Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial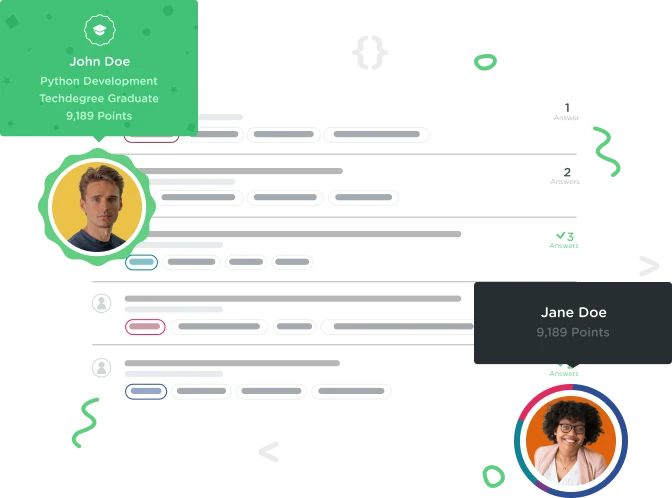

lydialavecchia
3,826 PointsImproving the form validation - booleans functions
Hi Team,
I have completed the challenge and the course but I think I am still confused about how the boolean functions affect the below code.
Would anybody be able to explain to me this as simply as possible?
Thanks a lot!
1 Answer

Erik Nuber
20,629 PointsBreaking it all down by sections...
Here you are defining the variables the first two variables are making it a little easier to type elsewhere. Instead of having to type $("#password") all the time, you are cutting down what you type and, as it is holding a jQuery ID Andrew suggests using the $ before your variable name itself. I am unsure though if this is standard or not. The next part simply starts the span tags as being hidden.
// problem: hints are shown even form is valid
// solution : show hints just when required
var $password = $("#password");
var $confirmPassword = $("#confirm_password");
//hide the hints
$("form span").hide();
These are simple functions that check for certain things. The first checks the length of the password and returns true if the length of the password is greater than 8 and false if less than or equal to 8. The next checks to see if the password and the confirmation password are equal. This is why the val() is used. It grabs the value of each variable and checks it. Again, we return true if they are equal or false if they are not equal. The final function here calls these functions to check and see if these three statements are true or not. I don't see a function in your js file for userNamePresent() though? However, if all of these statements are true, we return true and the submit button will become clickable, else false is returned and keeps the submit button from being able to be clicked.
function isPasswordValid() {
return $password.val().length > 8;
}
function arePasswordMatching(){
return $password.val() === $confirmPassword.val();
}
function canSubmit(){
return isPasswordValid() && arePasswordMatching() && userNamePresent();
}
These two functions are checking the validity of both password and confirm password. In the first function, it asks if the isPasswordValid() which grabs the function above and a true or false is returned. If true is returned the hint is hidden if not the hint is shown. The next() is used because the span is the child of password.
The confirmPasswordEvent() function is used to check if both password and confirm password are the same. If they are, the hint on the confirm password is hidden. Again, the next() is used because the span is the child of the $confirmPassword. If they are not the same than the hint is shown.
function passwordEvent(){
//find out if password is valid
if(isPasswordValid()){
//hide hint if valid
$password.next().hide();
} else {
//else show hint
$password.next().show();
}
}
function confirmPasswordEvent(){
//find out is password and confirmation match
if(arePasswordMatching()){
//hide hint if matched
$confirmPassword.next().hide();
} else {
//else show hint
$confirmPassword.next().show();
}
}
This function is grabbing the submit button. It is changing the property of the that button to disabled based on the canSubmit() function. Remember that the ! is a not operator so true is actually false and false is actually true. So initially we can't submit the button because canSubmit() is false which sets disabled to true and, when the information is correct we can canSubmit is true so disabled is then set to false and we are able to click the button. This might be where the most confusion is because of that not operator.
function enableSubmitEvent(){
$("#submit").prop("disabled", !canSubmit())
}
These two are controlling when the functions are being called for password event and the submit button events happen. In other words, they are calling the functions when focus or the pressed key is going from pressed down to it's normal up position.
//when event happens of password input
$password.focus(passwordEvent).keyup(passwordEvent).keyup(enableSubmitEvent);
// when event happens on a confirmation input
$confirmPassword.focus(confirmPasswordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent);
This calls the function for the submit button to actually happen when the document is ready to go. This is what starts the submit button functionality
enableSubmitEvent();
lydialavecchia
3,826 Pointslydialavecchia
3,826 PointsThank you so much Erik, for your thorough answer. This makes more more sense :)