Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial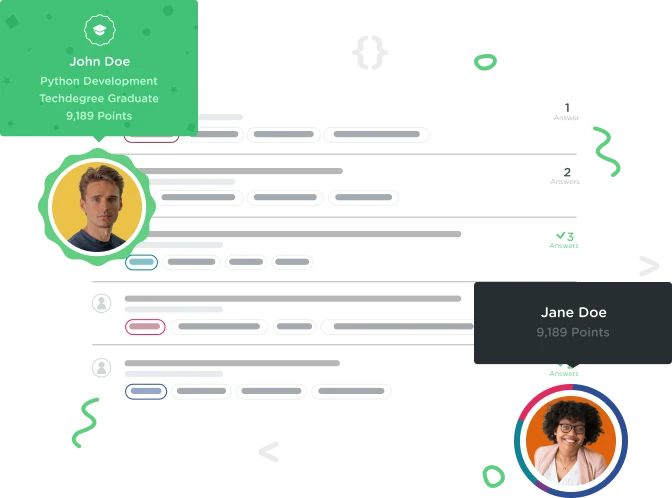
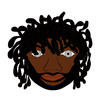
SAMUEL LAWRENCE
Courses Plus Student 8,447 PointsImproving the random number generator challenge with functions.
Here is my solution for this challenge. I had to copy the method of how to generate a random number between two numbers because I don't understand the math.
Math.random();
generates a random number between 0 and 1 but not including 1. Guil Hernandez said to get it to include up to 1 you add 1.
Math.random() + 1;
to get it to generate a random number between 1 and 6 you multiply by 6 then add 1
Math.random() * 6 + 1;
to get it to generate a number between two numbers it's
Math.random() * (6 -1 + 1) + 1;
where 6 is equal to the upper number and the minus 1 is equal to the lower number and you add 1 then you add back the lower number so this code could be rewritten this way.
Math.random() * (8 - 4 + 1) + 4;
this would generate a random number between 4 and 8. but I don't understand that math.
Anyway once I got that it was easy to figure out the rest. here is my solution.
function getRandomNumber ( lower, upper) {
var randomNumber = Math.floor(Math.random() * (parseInt(upper) - parseInt(lower) + 1)) + parseInt(lower);
return randomNumber;
}
document.write( getRandomNumber(0, 6) );
console.log( getRandomNumber(0, 10) );
A lot less code than the first random number game. Just for fun I went ahead and changed it so it would accept user input. Please provide some feedback. thanks
function getRandomNumber ( lower, upper) {
var randomNumber = Math.floor(Math.random() * (parseInt(upper) - parseInt(lower) + 1)) + parseInt(lower);
return randomNumber;
}
var lower = prompt('Type a number.');
var upper = prompt('Type a number higher than the number you just typed.');
document.write( getRandomNumber(lower, upper ) );
1 Answer
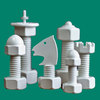
Steven Parker
231,275 PointsIt might help to think of the random formula as: Math.floor( Math.random() * range ) + minimum, where the "range" is one more than the difference between the maximum and the minimum.
Also, you wouldn't need parseInt in the function if the arguments passed in are already numbers.
SAMUEL LAWRENCE
Courses Plus Student 8,447 PointsSAMUEL LAWRENCE
Courses Plus Student 8,447 PointsThank you Steven Parker I will keep that in mind. I didn't notice that.