Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial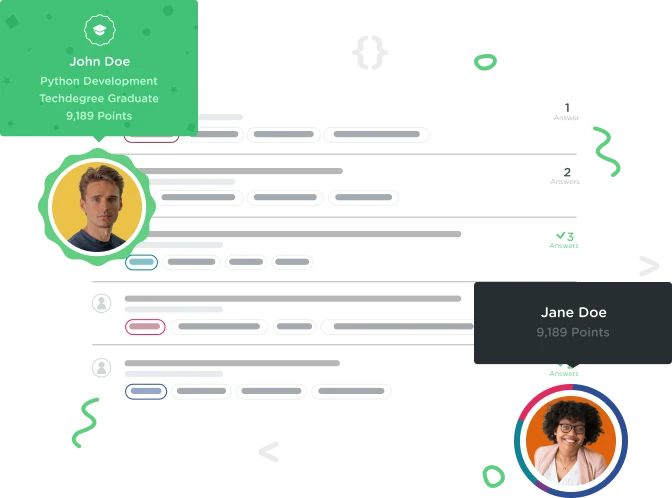

jaysonmena
12,539 PointsIn a string, how do I make correct indefinite articles in a story where the nouns are entered by the user?
In the Story Maker exercise, I used the prompt command to have the user type in a noun to work in my story. My story makes use of indefinite articles, e.g., "Once upon a time there was a..." What code could I write that would apply a rule about the use of indefinite articles so that if someone chooses the noun "apple," the definite article would output as "an" ?
1 Answer
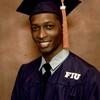
Dane Parchment
Treehouse Moderator 11,075 PointsThere are many different ways you can go about doing this, most of which I believe are outside the scope of what you are doing. Here I will give you some examples of what you are trying to accomplish.
Using if statements and OR logic: What we do here is ask the user for a noun, we then use the substring method, to grab the first letter of the string the user types in, if that string is a vowel, then we will print out "an + the variable" otherwise print out "a + the variable".
var noun = prompt("Please enter a noun", "enter noun here");
var firstLetter = noun.substring(0, 1);
if(firstLetter === "a" || firstLetter === "e" || firstLetter === "i" || firstLetter === "o" || firstLetter === "u") {
document.write("an " + noun);
}
else {
document.write("a " + noun);
}
Using arrays: Here we specify an array of vowels, we then ask the user for a noun, using the substring method we grab the first letter, and check and see if it that letter is in the array by using the includes method for the array. If it is included, print out "an + variable" otherwise print "a + variable".
var nounArray = ['a', 'e', 'i', 'o', 'u'];
var noun = prompt("Please enter a noun.", "Enter a noun here");
var firstLetter = noun.substring(0, 1);
if(nounArray.includes(firstLetter)) {
document.write("an " + noun);
}
else {
document.write("a " + noun);
}
Both of these work, but I would use the array because it is more easily expandable. There are much better solution, (I would use functions and stuff) but its early, I am in class, and I think these answers will suffice for now. If you still need help let me know.
I am expecting you to be able to find a way to implement these into your program, but if you need help let me know.

jaysonmena
12,539 PointsMuch thanks.

Michael Norelli
1,373 PointsThis was an interesting challenge. Here's what I did, using the invitation in the lesson to check out the Mozilla Developer Network. On that page, there is a method called ".search()" that works perfectly in this case:
if (noun1[0].search("[aeiou]") === -1) {
var article = "a";
} else var article = "an";
This will search the first letter ([0]
) of the string in the variable noun1
using a regular expression to see if it is a vowel ([aeiou]
).
If it is NOT a vowel, the first part of the if
statement is executed, setting a new global variable article
to "a".
If it is a vowel, it will set article
to "an" instead.
You can then concatenate article
alongside noun1
to make your story make sense.
john larson
16,594 Pointsjohn larson
16,594 PointsDane and Michael, you both gave very insightful answers. I had gone through documentation on search() before, and it had never occurred to me to use it in a situation like this. Very clever. Of the answers, search seemed most concise to me. But both answers expand my realization that the language is just the language, it's up to us to find ways to apply it.