Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial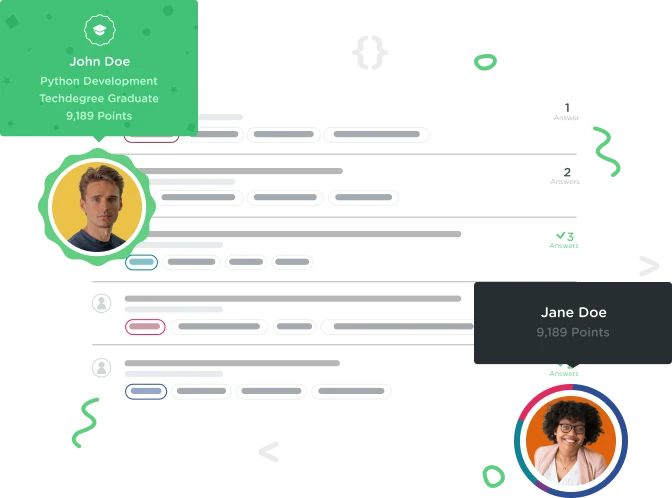
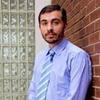
Zach Freitag
20,341 PointsIn Blog.java add a new method called getCategoryCounts. It should return a Map of category-to-count calculated by loopin
Solution?
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
}
1 Answer

Yanuar Prakoso
15,196 PointsHi Zach
This is my proposed solution. Please read also the comments inside:
//before this do not forget to import : java.util.Map; and java.util.HashMap;
public Map<String,Integer> getCategoryCounts(){
//intializing the map:
Map<String,Integer> categoryCounter = new HashMap<>();
//iterate all post in the List of mPosts to obtain the category for each post:
for(BlogPost post : mPosts){
//fetch the category of the post:
String category = post.getCategory();
//if the category is new (has not been counted yet) then initialize new counter for it:
if(categoryCounter.get(category)==null){
int counter = 0;
//map the category to the newly initialized counter:
categoryCounter.put(category, counter);
}
//if the category alredy has counter specified for it, we just fetch it from the map:
int counter = categoryCounter.get(category);
//then we increment the category's counter:
counter++;
//put it back to the map:
categoryCounter.put(category, counter);
}
//when we're done iterating all of mPosts:
return categoryCounter;
}
PS: You need to use Map<String,Integer> not Map<String,int> because you can't use primitive type (int) as generic argument in Java more on this and also if you need reference on using interface Map in Java and class HashMap in Java
I hope this can help a little