Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial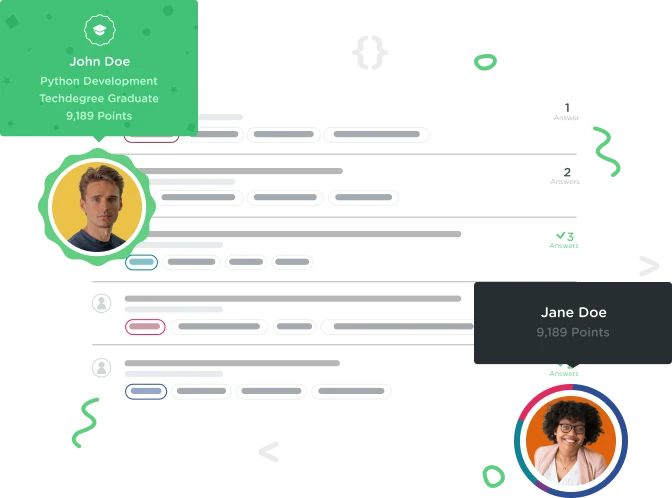
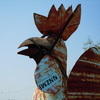
Will Anderson
28,683 PointsIn ComicBookGalleryModel, adding ComicBookArtist class + changes results in numerous build errors - Help plz!
Added ComicBookArtist.cs followed with changes to Artist.cs and ComicBook.cs, get 'Inconsistent accessibility' warnings resulting in 10 build errors.
Errors dump:
Severity Code Description Project File Line Suppression State
Error CS0053 Inconsistent accessibility: property type 'ICollection<ComicBookArtist>' is less accessible than property 'Artist.ComicBooks' ComicBookGalleryModel C:\Users\kanderson321\documents\visual studio 2017\Projects\ComicBookGalleryModel\ComicBookGalleryModel\Models\Artist.cs 20 Active
Error CS0053 Inconsistent accessibility: property type 'ICollection<ComicBookArtist>' is less accessible than property 'ComicBook.Artists' ComicBookGalleryModel C:\Users\kanderson321\documents\visual studio 2017\Projects\ComicBookGalleryModel\ComicBookGalleryModel\Models\ComicBook.cs 26 Active
Error CS0051 Inconsistent accessibility: parameter type 'Role' is less accessible than method 'ComicBook.AddArtist(Artist, Role)' ComicBookGalleryModel C:\Users\kanderson321\documents\visual studio 2017\Projects\ComicBookGalleryModel\ComicBookGalleryModel\Models\ComicBook.cs 36 Active
Error CS1503 Argument 1: cannot convert from 'ComicBookGalleryModel.Models.Artist' to 'ComicBookGalleryModel.Models.ComicBookArtist' ComicBookGalleryModel C:\Users\kanderson321\documents\visual studio 2017\Projects\ComicBookGalleryModel\ComicBookGalleryModel\Program.cs 55 Active
Error CS1503 Argument 1: cannot convert from 'ComicBookGalleryModel.Models.Artist' to 'ComicBookGalleryModel.Models.ComicBookArtist' ComicBookGalleryModel C:\Users\kanderson321\documents\visual studio 2017\Projects\ComicBookGalleryModel\ComicBookGalleryModel\Program.cs 56 Active
Error CS1503 Argument 1: cannot convert from 'ComicBookGalleryModel.Models.Artist' to 'ComicBookGalleryModel.Models.ComicBookArtist' ComicBookGalleryModel C:\Users\kanderson321\documents\visual studio 2017\Projects\ComicBookGalleryModel\ComicBookGalleryModel\Program.cs 64 Active
Error CS1503 Argument 1: cannot convert from 'ComicBookGalleryModel.Models.Artist' to 'ComicBookGalleryModel.Models.ComicBookArtist' ComicBookGalleryModel C:\Users\kanderson321\documents\visual studio 2017\Projects\ComicBookGalleryModel\ComicBookGalleryModel\Program.cs 65 Active
Error CS1503 Argument 1: cannot convert from 'ComicBookGalleryModel.Models.Artist' to 'ComicBookGalleryModel.Models.ComicBookArtist' ComicBookGalleryModel C:\Users\kanderson321\documents\visual studio 2017\Projects\ComicBookGalleryModel\ComicBookGalleryModel\Program.cs 73 Active
Error CS1503 Argument 1: cannot convert from 'ComicBookGalleryModel.Models.Artist' to 'ComicBookGalleryModel.Models.ComicBookArtist' ComicBookGalleryModel C:\Users\kanderson321\documents\visual studio 2017\Projects\ComicBookGalleryModel\ComicBookGalleryModel\Program.cs 74 Active
Error CS1061 'ComicBookArtist' does not contain a definition for 'Name' and no extension method 'Name' accepting a first argument of type 'ComicBookArtist' could be found (are you missing a using directive or an assembly reference?) ComicBookGalleryModel C:\Users\kanderson321\documents\visual studio 2017\Projects\ComicBookGalleryModel\ComicBookGalleryModel\Program.cs 88 Active
ComicBookArtist.cs:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ComicBookGalleryModel.Models
{
class ComicBookArtist
{
public int Id { get; set; }
public int ComicBookId { get; set; }
public int ArtistId { get; set; }
public int RoleId { get; set; }
public ComicBook ComicBook { get; set; }
public Artist Artist { get; set; }
public Role Role { get; set; }
}
}
Artist.cs: (ComicBooks in line 20 is the error)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ComicBookGalleryModel.Models
{
public class Artist
{
public Artist()
{
ComicBooks = new List<ComicBookArtist>();
}
public int Id { get; set; }
public string Name { get; set; }
public ICollection<ComicBookArtist> ComicBooks { get; set; }
}
}
ComicBook.cs: (Artists in line 26, AddArtist(Artist artist, Role role) in line 36 are errors)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data.Entity;
namespace ComicBookGalleryModel.Models
{
public class ComicBook
{
public ComicBook()
{
Artists = new List<ComicBookArtist>();
}
public int Id { get; set; }
public int SeriesId { get; set; }
public int IssueNumber { get; set; }
public string Description { get; set; }
public DateTime PublishedOn { get; set; }
public decimal? AverageRating { get; set; }
public Series Series { get; set; }
public ICollection<ComicBookArtist> Artists { get; set; }
public string DisplayText
{
get
{
return $"{Series?.Title} #{IssueNumber}";
}
}
public void AddArtist(Artist artist, Role role)
{
Artists.Add(new ComicBookArtist()
{
Artist = artist,
Role = role
});
}
}
}
I can't continue until I find a way to build this solution 8-|
1 Answer

James Churchill
Treehouse TeacherWill,
You need to add a public
access modifier to your ComicBookArtist class.
public class ComicBookArtist
{
...
}
Once you've made that change, it looks like you'll still have other errors to resolve, but hopefully this gets you unstuck.
Thanks ~James