Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial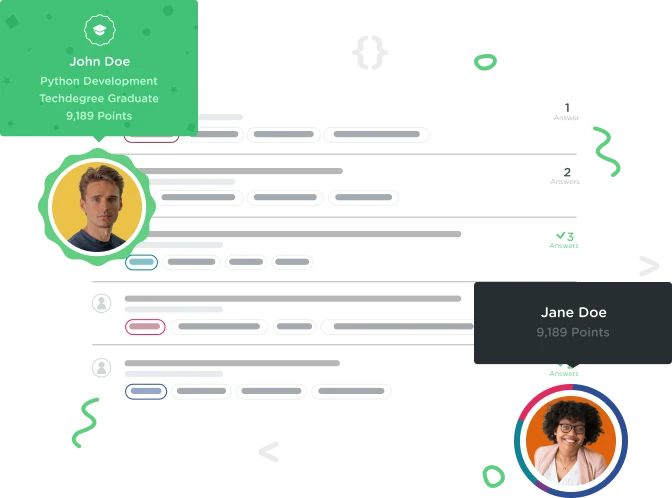
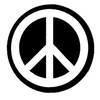
john larson
16,594 PointsIn his teachers notes Kenneth says "Just take out the string formatting for that error message"... but how?
The error happens when a non integer is entered into the input field. This code is the teachers code downloaded from Treehouse.
try:
# get a number guess from the player
guess = int(input("Guess a number between 1 and 10: "))
except ValueError:
print("{} isn't a number!".format(guess))
else:
this gets printed to the console
Guess a number between 1 and 10: one
Traceback (most recent call last):
File "C:\Users\smolderingflax\Python Basics\teachers_num_game.py", line 12, in game
guess = int(input("Guess a number between 1 and 10: "))
ValueError: invalid literal for int() with base 10: 'one'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "C:\Users\smolderingflax\Python Basics\teachers_num_game.py", line 33, in <module>
game()
File "C:\Users\smolderingflax\Python Basics\teachers_num_game.py", line 14, in game
print("{} isn't a number!".format(guess))
UnboundLocalError: local variable 'guess' referenced before assignment
This causes the program to exit instead of the exception being handled and the program continuing
2 Answers

Sabine Maennel
Treehouse Project ReviewerHello John,
in your code segment:
try:
# get a number guess from the player
guess = int(input("Guess a number between 1 and 10: "))
except ValueError:
print("{} isn't a number!".format(guess))
else:
When you print the error: guess doesn't even exist and you try to use i in your format statement for the error. I don't remember the lesson exactly, but this is what I would do:
# get a number guess from the player
guess = input("Guess a number between 1 and 10: ")
try:
guess = int(guess)
except ValueError:
print("{} isn't a number!".format(guess))
When you do it that way: the input statement is save, since you make no assumption, whether it is a number or not. Then the guess = int(guess) statement is unsafe and therefore you put that into a try-except-clause. That way you can react if it fails and now your print statement contains the original guess, which was also safe.
Did this answer help you? With kind regards, Sabine
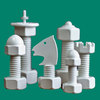
Steven Parker
231,269 PointsSabine's solution is a bit different from (and possibly better than ) the instructor's suggestion.
I believe what Kenneth was suggesting would look something like this:
try:
# get a number guess from the player
guess = int(input("Guess a number between 1 and 10: "))
except ValueError:
print("That isn't a number!")
else:
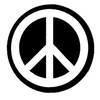
john larson
16,594 PointsHi Steven, leaving out the variable part is one of the suggestions (I think yours actually) I still get the error message with that. The BIG error message not the exception error message that was from the try: except.
john larson
16,594 Pointsjohn larson
16,594 PointsI think I TRIED to try this approach before but I missed that the input needs to go OUTSIDE the try: