Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial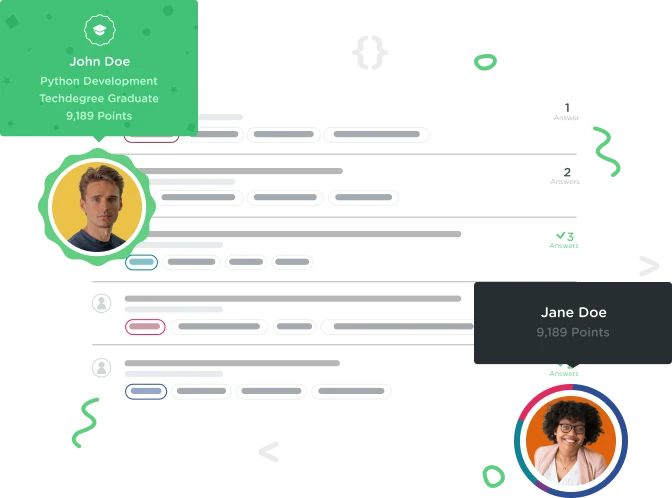

Jami Schwarzwalder
17,961 PointsIn Java, What is the difference between Var.function and function(var)
I keep getting lost on when I should be dotting into a variable and when to pass it through.
I think this is one of the biggest problems I"m having when writing Java.
3 Answers

Nikolay Mihaylov
2,810 PointsI am not sure I understand you question correct but here it goes.
Lets say we have a variable name of the class String which which holds the value John Smith.
String name = "John Smith";
The String class provides a number of methods to manipulate its content. For example the toLowerCase() method which converts all capital letters to lowercase. When you are trying to access this functionality ( the lowercase method of the String object name ) from another object, like this:
public class Test {
public static void main(String[] args) {
String name = "John Smith";
System.out.println( name.toLowerCase() );
}
}
You need to use the '.' operator to access any variables and methods of the name object. So basicly when the jvm reads the compiled code it does something like that:
public class Test {
public static void main(String[] args) {
String name = "John Smith";
System.out.println(
name // Find the object with a reference name
. // access the object
toLowerCase() // call the method inside the object
);
}
}
Now lets take a look at another method called subString() which is also a part of the String class.
public class Test {
public static void main(String[] args) {
String name = "John Smith";
System.out.println( name.subString(2) );
}
}
What it does is: Returns a new string that is a substring of this string. The substring begins with the character at the specified index and extends to the end of this string. from the Java API doc.
So this will output -> hn Smith
Some functins require some information to be passed in order for them to be able to do their job. For example the subString function returns the text in the name variable without the first two letters. However when you call it doesn't know which letters to cut out so it asks you for an argument.
public class Test {
public static void main(String[] args) {
String name = "John Smith";
System.out.println(
name // Find the object with a reference name
. // access the object
subString(2) // call the method inside the object and give it a value for its int argument.
);
}
}
You could also check this article. Hope it helps and I hope I understood your question correct.

Craig Dennis
Treehouse TeacherHi Jami!
I might be able to help if you give me an example, I'm having a hard time picturing the loose function you are encountering.
Java is Object Oriented and very heavy on classes. Almost everything you see that is callable (uses ()) is a method, which means it belongs to the instance you are working on.
So an instance of a string has a method like this:
"hello".length();
// and also has methods that take arguments like this:
"hello".equals("goodbye");
Not sure if that cleared anything up, but I hope it did. Do please provide a specific example and I'll see if we can't dissect the problem ;)

Jami Schwarzwalder
17,961 PointsI struggle to know when to put things before the period or in the parentheses. I just got this unhelpful error
./Example.java:7: error: cannot find symbol System.out.printf(getColor(goKart)); ^ symbol: method getColor(GoKart) location: class Example 1 error
public class Example {
public static void main(String[] args) {
System.out.println("We are going to create a GoKart");
GoKart goKart = new GoKart("blue");
System.out.printf(getColor(goKart));
}
}
I'm starting to think, that error means that goKart needs to come before getColor, but when there are multiple variables and functions in one line, I can't figure out which one goes in the parenthesis and which one comes before.

Nikolay Mihaylov
2,810 PointsYou are correct in this case. It should be
public class Example {
public static void main(String[] args) {
System.out.println("We are going to create a GoKart");
GoKart goKart = new GoKart("blue");
System.out.printf(goKart.getColor());
}
}
This is because the getColor() method is part of the GoKart class. So you have to use the dot operator in order to access the goKart object. You get this error because of that. Since you are not accessing any object with the dot operator the program assumes that the getColor() method belongs to the Example class. However the example class doesn't have this kind of method. Therefor java gives an error saying that it can't find the method getColor() inside the Example class. This error message is actually on the better side of things when it comes to how helpfull error message really are.