Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial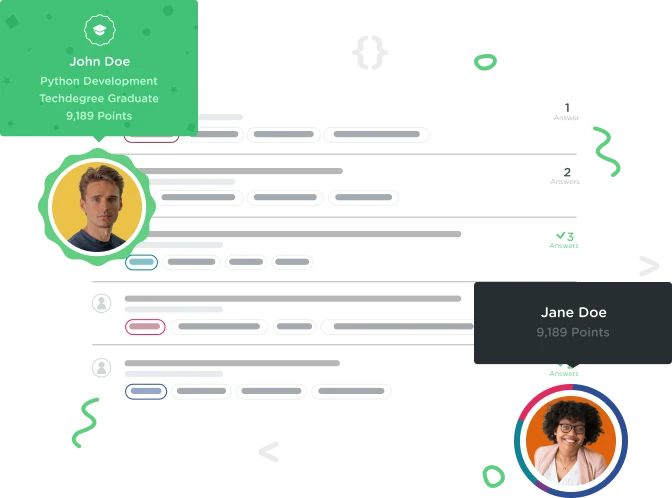

sahil jagdale
Courses Plus Student 1,043 Pointsin letter guessing game, everything is perfect ,still after guessing every letter correct it still asks for letter
its like infinite loop
import random
words = ['apple','mango','banana','strawberry','blueberry','lemon','lime','watermelon'] while True: start = input("press enter to start , or enter Q/q to quit") if start.lower() == 'q': break secret_word = random.choice(words) bad_guesses = [] good_guesses = []
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print('')
print("strikes: {}/7".format(len(bad_guesses)))
print('')
guess = input("guess a letter: ").lower()
if len(guess) != 1:
print("please guess letter by letter one at a time! assole")
continue
elif not guess.isalpha():
print("its not a number guessing game assole! guess the alphabet")
continue
elif guess in bad_guesses or guess in good_guesses:
print("you have already guessed that letter!" )
continue
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("you win!! word was {} ".format(secret_word))
break
else:
bad_guesses.append(guess)
else:
print("you didnt guessed it! my secret word was {} .".format(secret_word))
output:
guess a letter: o
watermelo_
strikes: 2/7
guess a letter: n
watermelon
strikes: 2/7
guess a letter:
2 Answers

Gavin Ralston
28,770 Pointswhile len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word))
So this reads "While the length of bad_guesses is less than seven and the length of good_guesses is less than the length of the characters in the secret_word" keep prompting for guesses.
But there's no end condition that can be reached if you have multiple copies of the same letter in the word right now:
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("you win!! word was {} ".format(secret_word))
break
So if you picked "watermelon" and you picked the letter 'e' you'd increase the number of good guesses by one, but you'd fill two blank spaces in the word. You could never pick it again to increase the "good guess" count, either. So you're kind of stuck right now unless the word contains all unique letters.
Maybe there's another way to verify you've picked all the letters in the set of characters for that word, or another way to keep track of the count?

Endrin Tushe
4,591 PointsI think the problem may have been that the variables: secret_word, good_guesses, and bad_guesses were defined after the start game input. I re-ordered those three variables and tried your code again and everything works okay. When you get to the end if you don't get the word correctly you get prompted by the start prompt to enter or quit the game. Here's your code again, it should work okay:
'''python
import random
words = ['apple','mango','banana','strawberry','blueberry','lemon','lime','watermelon']
while True:
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
start = input("press enter to start , or enter Q/q to quit")
if start.lower() == 'q':
break
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print('')
print("strikes: {}/7".format(len(bad_guesses)))
print('')
guess = input("guess a letter: ").lower()
if len(guess) != 1:
print("please guess letter by letter one at a time! assole")
continue
elif not guess.isalpha():
print("its not a number guessing game assole! guess the alphabet")
continue
elif guess in bad_guesses or guess in good_guesses:
print("you have already guessed that letter!" )
continue
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("you win!! word was {} ".format(secret_word))
break
else:
bad_guesses.append(guess)
else:
print("you didnt guessed it! my secret word was {} .".format(secret_word))'''

Gavin Ralston
28,770 PointsGood catch!
I tried quickly answering this but didn't catch it was in a while loop.
He's still got to account for the fact that any letter only gets added once to the good_guesses list when he guesses correctly, though. If it only gets added once to the good_guess list, words like "strawberry" or "peel" or "too" won't ever have a long enough length to reach the win condition, since there is input validation on the letters to make sure he doesn't guess the same one twice.