Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial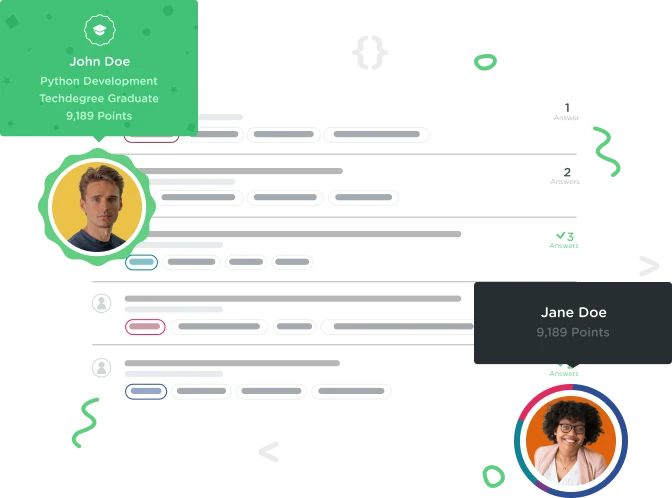

Nafeez Quraishi
12,416 Pointsin `<main>': undefined method `push' for nil:NilClass (NoMethodError)
Having a hard time to identify what i am not doing right here. My code is almost a copy of what is given in the example:
require "./Contact"
class AddressBook
attr_accessor :contacts
def intitialize
@contacts = []
end
def print_contact_list
contacts.each do |contact|
puts contact.to_s('last_first')
end
end
end
me = Contact.new
me.first_name = "AAA"
me.middle_name = "BBB"
me.last_name = "CCC"
me.add_phone_number('first_no', '111111111')
me.add_phone_number('second_no', '1111122222')
me.add_address('Home', "my house", 'my street one', 'my city', "my state", "999999")
me.add_address('Office', 'my office', 'my office street', 'my city', "my state", '9999111')
my_frnd = Contact.new
my_frnd.first_name = "DDD"
my_frnd.middle_name = "EEE"
my_frnd.last_name = "FFF"
my_frnd.add_address('Home', "frnd house", 'frnd street one', 'frnd city', "frnd state", "999999")
my_frnd.add_address('Office', 'frnd office', 'frnd office street', 'frnd city', "frnd state", '9999111')
address_book = AddressBook.new
address_book.contacts.push(me)
address_book.contacts.push(my_frnd)
address_book.print_contacts_list
2 Answers

Nafeez Quraishi
12,416 PointsMade contacts a global variable i.e. $contacts and that worked.
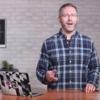
Jay McGavren
Treehouse TeacherNafeez Quraishi sorry you didn't get an answer on this sooner!
I would not recommend using global variables. They eliminate "encapsulation", meaning that a bug in one part of your program can cause problems that show up in a completely different part of your program, and you will have a very hard time finding the real source of the problem. Instead, we should try to fix the real source of this error.
It looks like the main problem is a simple typo - the name of the initialize
method was misspelled, and so @contacts
wasn't getting initialized. My updates to your code are below, with comments.
# I changed this to lower-case to match the name of the "contact.rb" file.
require "./contact"
class AddressBook
attr_accessor :contacts
# This here was the main problem.
# You had a typo "intitialize", in the name of this method. So when you
# called AddressBook.new, Ruby looked for a method named "initialize",
# saw that there wasn't one, and did nothing. That's why you were getting
# "undefined method 'push' for nil:NilClass": @contacts was still set to
# nil.
def initialize
@contacts = []
end
def print_contact_list
contacts.each do |contact|
puts contact.to_s('last_first')
end
end
end
me = Contact.new
me.first_name = "AAA"
me.middle_name = "BBB"
me.last_name = "CCC"
me.add_phone_number('first_no', '111111111')
me.add_phone_number('second_no', '1111122222')
me.add_address('Home', "my house", 'my street one', 'my city', "my state", "999999")
me.add_address('Office', 'my office', 'my office street', 'my city', "my state", '9999111')
my_frnd = Contact.new
my_frnd.first_name = "DDD"
my_frnd.middle_name = "EEE"
my_frnd.last_name = "FFF"
my_frnd.add_address('Home', "frnd house", 'frnd street one', 'frnd city', "frnd state", "999999")
my_frnd.add_address('Office', 'frnd office', 'frnd office street', 'frnd city', "frnd state", '9999111')
address_book = AddressBook.new
address_book.contacts.push(me)
address_book.contacts.push(my_frnd)
# Fixed a typo in the method name here.
address_book.print_contact_list