Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial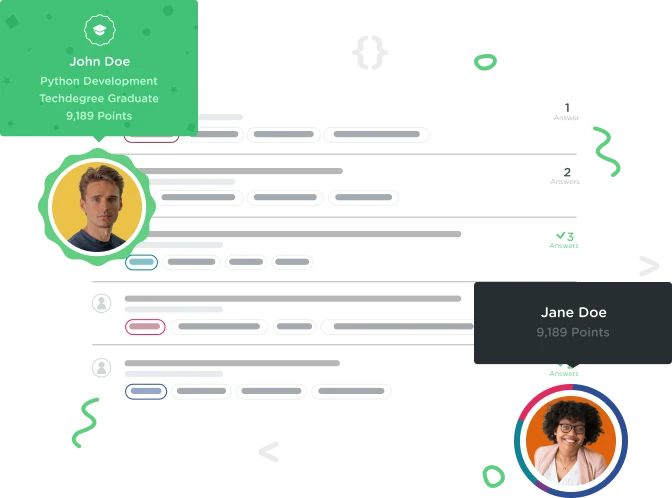

Hafeez Khan
1,270 PointsIn my FunFactsActivity class, i keep getting three errors, all three of them being, cannot resolve symbol "R".
public class FunFactsActivity extends Activity {
private FactBook mFactBook = new FactBook();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Declare our View Variables and assign the Views from the layout file
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.ShowFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
String fact = mFactBook.getFact();
//Update the label with our dynamic fact
factLabel.setText(fact);
}
};
showFactButton.setOnClickListener(listener);
}
}
Everything above is my current code, the errors are occurring where ever the "R" is, PLEASE HELP.

Hafeez Khan
1,270 Points<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".FunFactsActivity" android:background="#ff56e07e">
<TextView
android:text="Did You Know?"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="24sp"
android:textColor="#80ffffff"
android:id="@+id/"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true" />
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Show Another Fun Fact"
android:id="@+id/showFactButton"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:background="@android:color/white"
android:textColor="@android:color/background_dark" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="The fastest slapshot on record is Bobby Hull's, which registered one hundred and eighteen miles per hour, almost 10 miles faster the Chara"
android:id="@+id/factTextView"
android:layout_centerVertical="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:textSize="24sp"
android:textColor="@android:color/white" />
</RelativeLayout>
Everything above is my activity_fun_facts.xml
2 Answers

justindennison
15,922 Pointspublic class FunFactsActivity extends Activity {
private FactBook mFactBook = new FactBook();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Declare our View Variables and assign the Views from the layout file
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById**(R.id.ShowFactButton)**;
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
String fact = mFactBook.getFact();
//Update the label with our dynamic fact
factLabel.setText(fact);
}
};
showFactButton.setOnClickListener(listener);
}
}
Take a look at the bolded section of your Java code. Notice that you have
android:id="@+id/showFactButton"
which shows that you have an id that is lower CamelCase but in your java code uses the capital version. Fixing that may help out.
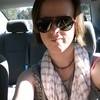
karis hutcheson
7,036 PointsI'm guessing from the error that you're missing an import declaration. If you look at your code in the IDE (assuming you're using Android Studio), click on "R" and press alt+return, it should give you a solution or the option to import the correct class.
justindennison
15,922 Pointsjustindennison
15,922 PointsTaking a look at your code, my first instinct is that
Button showFactButton = (Button) findViewById(R.id.ShowFactButton);
should be
Button showFactButton = (Button) findViewById(R.id.showFactButton);
That may not be the error if you have set the button's id to
ShowFactButton
I have a follow up question so maybe I can be of some more help, do you have all of those elements added to your layout using either the graphical layout tool or the XML design? If so, could you send your XML layout contents that are inside of activity_fun_facts.xml?