Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial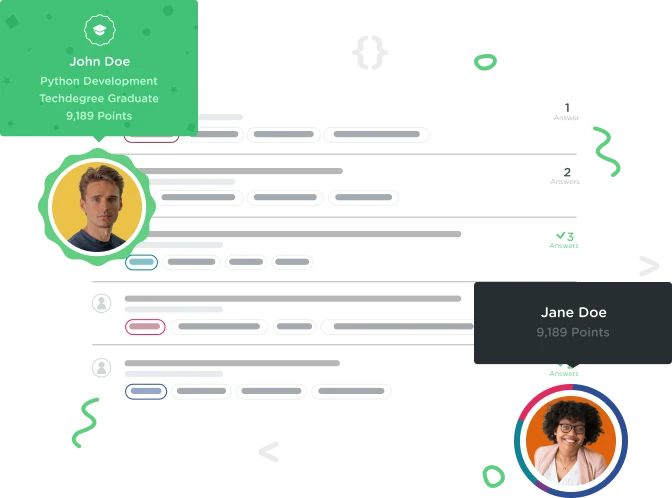
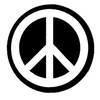
john larson
16,594 PointsIn my own editor this returns Jason Seifer, the challenge editor says it returns Kenneth Love
So it's not passing. Any thoughts?
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
teachers_dict = {
'Jason Seifer': [
'Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': [
'Python Basics', 'Python Collections']
}
jason = []
kenneth = []
def most_classes(teachers_dict):
for key in teachers_dict:
if key == "Jason Seifer":
for course in teachers_dict[key]:
jason.append(course)
else:
for course in teachers_dict[key]:
kenneth.append(course)
if len(jason) > len(kenneth):
return "Jason Seifer"
else:
return "Kenneth Love"
result = most_classes(teachers_dict)
print(result)
1 Answer
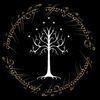
jacinator
11,936 PointsTry moving jason
and kenneth
into the function.
...
def most_classes(teachers_dict):
jason = []
kenneth = []
for key in teachers_dict:
if key == "Jason Seifer":
for course in teachers_dict[key]:
jason.append(course)
else:
for course in teachers_dict[key]:
kenneth.append(course)
if len(jason) > len(kenneth):
return "Jason Seifer"
else:
return "Kenneth Love"
result = most_classes(teachers_dict)
print(result)
john larson
16,594 Pointsjohn larson
16,594 PointsI just tried that, thank you... but it's still not passing. Jusy so II understand your thought process, What were you thinking that would do?
jacinator
11,936 Pointsjacinator
11,936 PointsMoving the variables into the function makes them part of the local scope. Since that is the only place that you are using them, it is the only place that you need them defined. Defining them somewhere else could result in problems when your code runs.
This is the code that I used to pass the challenge.
john larson
16,594 Pointsjohn larson
16,594 Pointsjohn larson
16,594 Pointsjohn larson
16,594 PointsYour code is 10 lines shorter than mine. I'm gonna try'n figure what you did. I don't think we covered .items() but it looks pretty handy. It puts the dict in a list in tuples?
jacinator
11,936 Pointsjacinator
11,936 PointsYou nailed what
dict.items()
does.