Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial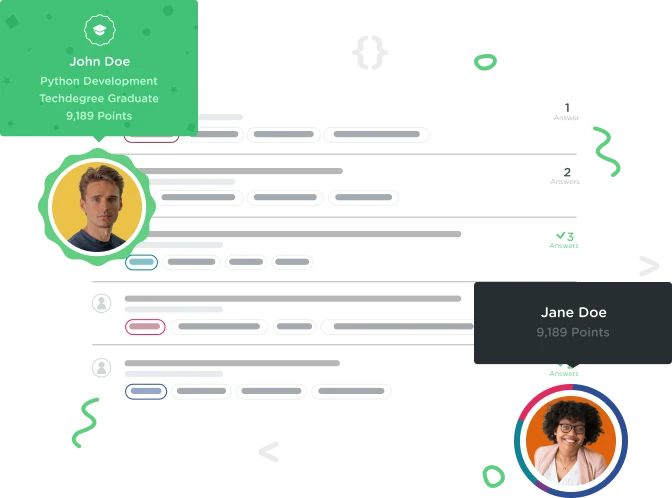

Boris Likhobabin
3,581 PointsIn need of further explanation of custom initializers!
While trying to finally get what initializers are for I came across this situation:
When I declare self.properties in the initializer instead of writing self.red = red or self.green = green I could simply write integers like self.red = 3 self.green = 5 and I it DOES NOT seem to affect the output (neither it gives me any errors in my playground). So my questions is what is the exact point of writing self.red = red and why don't I get any errors (or in which case I would get an error.)
(The code is correct and it passed, the only thing I changed is adding those integers like self.red = 3)
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = 2
self.green = 3
self.blue = 4
self.alpha = 5
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
let color = RGBColor(red: 10, green: 20, blue: 30, alpha: 35)
color.description

Boris Likhobabin
3,581 PointsBy this I mean that whether I have self.red = 2, self.green = 3..... or I have self.red = red, self. green = green.... color.description does not change I don't see any other way in which this change affects my struct and the instances created from it. (The only guess that I have in mind is that within struct self.red = 2 but (as I understand) struct is a blueprint so what I will really using is the instances created from it. so I don't see how this change affects the way new instances are created)
BTW thanks for such a fast reply!
2 Answers
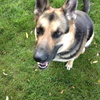
Eric Hodgins
29,207 Pointsok, I think I understand now. The reason the description property remains the same is because it's just simply using the variables that were put in for the color struct instance you made. Try using:
self.description = "red: \(self.red), green: \(self.green), blue: \(self.blue), alpha: \(self.alpha)"
You'll see I'm using the self keyword now. Let me know if that makes more sense or if you need further clarification!

Boris Likhobabin
3,581 PointsThanks a lot! I get it now =) Just as a follow up question what is then the difference between those two ways: the one with the self.red (like you suggested) and the one with just red. If it is the same then is there some sort of convention for which one to use?
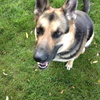
Eric Hodgins
29,207 PointsTypically "description" properties would refer to the properties of that particular instance. self.red refers to that particular instance's color. While red in this case is just the local variable passed in. Might make more sense if I rename things:
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(redColor: Double, greenColor: Double, blueColor: Double, alphaValue: Double) {
self.red = 2
self.green = 3
self.blue = 4
self.alpha = 5
//print out local parameters passed in
self.description = "red: \(redColor), green: \(greenColor), blue: \(blueColor), alpha: \(alphaValue)"
//print out the instance's properties
//self.description = "red: \(self.red), green: \(self.green), blue: \(self.blue), alpha: \(self.alpha)"
}
}
let color = RGBColor(redColor: 10, greenColor: 20, blueColor: 30, alphaValue: 35)
color.description
Also, note now that I've renamed the init's parameters the Swift compiler can now infer that you're referring to the instance and not the local variable. So to simplify things you could now write:
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
Eric Hodgins
29,207 PointsEric Hodgins
29,207 PointsWhat do you mean by it does not affect the output?