Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial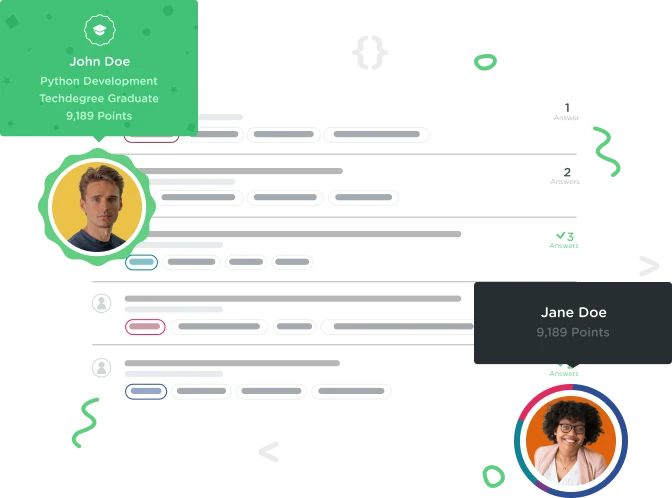

annecalija
9,031 PointsIn numstring.py, why is the implementation of __radd__ different from __add__?
Instead of
def __add__(self, other):
if '.' in self.value:
return float(self) + other
return int(self) + other
def __radd__(self, other):
return self + other
why not this?
def __add__(self, other):
if '.' in self.value:
return float(self) + other
return int(self) + other
def __radd__(self, other):
if '.' in self.value:
return float(self) + other
return int(self) + other
2 Answers

Marcin Lubke
13,253 PointsIf you do it like in the first example, than yes - __radd__
will call __add__
method "internally".
By typing float(self) + other
you call __add__
method and by typing other + float(self)
you call __radd__
method.
You could also try to do it another way - to write the whole code in __radd__
method, and inside __add__
call the second method by using other + float(self)
.

Marcin Lubke
13,253 PointsAs I understand, you can do it both ways, at least in this case.
Here, your __radd__
method simply returns __add__
method:
def __add__(self, other):
if '.' in self.value:
return float(self) + other
return int(self) + other
def __radd__(self, other):
return self + other
Here you just using another code for __radd__
method:
def __add__(self, other):
if '.' in self.value:
return float(self) + other
return int(self) + other
def __radd__(self, other):
if '.' in self.value:
return float(self) + other
return int(self) + other
Both ways are ok, but following DRY concept (don't repeat yourself) the first method should be better, it's a little shorter :)

annecalija
9,031 PointsHi Marcin,
Thanks for answering.
I am fully aware that both cases will work and yes, following DRY is recommended. My bad, I didn't explain clearly on what I really wanted to know from my question.
However, my confusion starts from why adding floats work on the __radd__
when it didn't even address adding floats like the one in __add__
. Is there a connection between how add and radd works (i.e. radd is calling add internally, idk)??
Could you kindly help me with this confusion? Thanks again in advance!
annecalija
9,031 Pointsannecalija
9,031 PointsHi Marcin,
Thank you so much! This gets clearer to me now.