Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial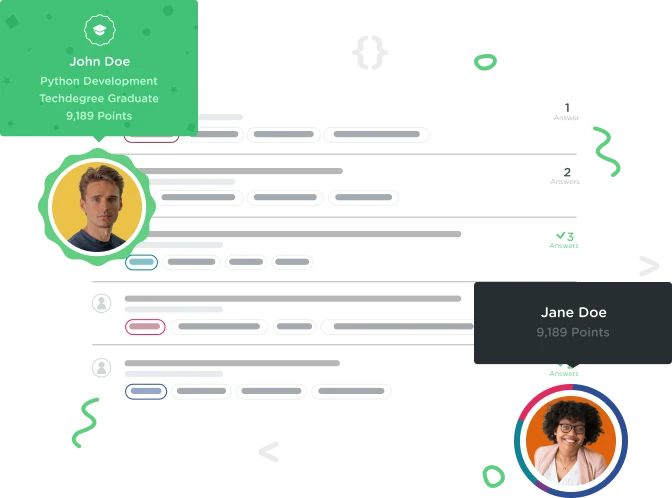

Jacob McQueen
2,166 PointsIn Objective C, whats the difference between Instance and Class methods, and how do you switch between the two?
I have been trying to get rid of an error, which is screen shotted here: http://i.imgur.com/RgvBLGh.jpg And Looking online someone said that they were able to fix the problem by changing their method from an instance method to a class method. So can someone explain to me how to do that? I don't know what the difference is.
1 Answer
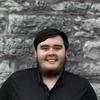
Michael Hulet
47,913 PointsAn instance method is a method that's only available on a particular instance of a class, only after said instance of the class has already been created. A class method is a method that can be called on the class itself, no instance necessary. For example:
YourClass *object = [[YourClass alloc] init];
[object someInstanceMethod];
The above is an instance method, because you must create an instance of YourClass
(in this case, the object
variable) before you can call the method "someInstanceMethod
". However, in a class method, you don't have to create the variable first, and you can simply call the method directly on the class name, like this:
[YourClass someClassMethod];
Instance methods are the most common type of method, because you'll most commonly be telling a specific object to perform some action. Class methods can be useful, however, when there's no contextual data needed in the method, and it can theoretically be implemented and run on any generic object. Class methods are common in convenience initializers, though. For example, instead of typing this:
NSObject *newObject = [[NSObject alloc] init];
you can type this to produce the same result:
NSObject *newObject = [NSObject new];
That can be done on any NSObject
or subclass thereof. In the above examples, both alloc
and new
are class methods, that both return a new instance of the NSObject
class. init
is an instance method, which sets up the new instance of NSObject
that alloc return
ed and subsequently return
s that. The new
class method invokes both of those for you and return
s the result.
Because of the difference in the way they work, class methods are declared a little differently than instance methods. Commonly, you'd declare and define an instance method like this:
@interface YourClass : NSObject
-(void)someInstanceMethod;
@end
#import "instance.h"
//There's some bad syntax highlighting by Treehouse's Markdown interpreter in this block. Ignore it, because the code works
@implementation YourClass
-(void)someInstanceMethod{
//Whatever you want your instance method to do here
}
@end
However, with a class method, you'd declare and define your method with a "+
", instead of a "-
", like this:
@interface YourClass : NSObject
+(void)someClassMethod;
@end
#import "class.h"
//Also bad syntax highlighting to be ignored here
@implementation YourClass
+(void)someClassMethod{
//Whatever you want your class method to do here, just like in an instance method
}
@end
Jacob McQueen
2,166 PointsJacob McQueen
2,166 PointsThank you. As far as my error I don't understand it, but I retyped in exactly what I had already typed and then it worked. Go figure.
Michael Hulet
47,913 PointsMichael Hulet
47,913 PointsGlad to hear it helped!