Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial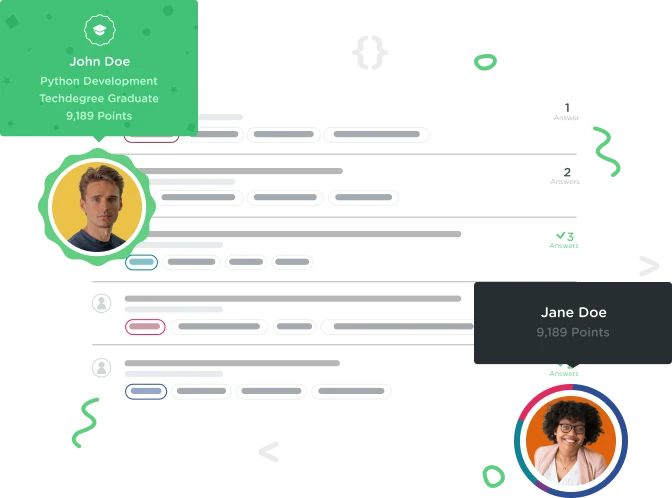
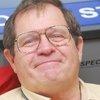
Joe Mackey
20,418 PointsIn Repository.cs update the AddCourse method to add a course to the context. Instantiate an instance of the
Not sure what I am doing wrong
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class Repository
{
public static List<Course> GetCourses()
{
using (var context = new Context())
{
return context.Courses
.OrderBy(c => c.Teacher.LastName)
.ThenBy(c => c.Teacher.FirstName)
.ToList();
}
}
public static List<Course> GetCoursesByTeacher(string lastName)
{
using (var context = new Context())
{
return context.Courses
.Where(c => c.Teacher.LastName == lastName)
.ToList();
}
}
public static Course GetCourse(int id)
{
using (var context = new Context())
{
return context.Courses
.Include(c => c.Teacher)
.SingleOrDefault(c => c.Id == id);In Repository.cs update the AddCourse method to add a course to the context.
Instantiate an instance of the Context class within a using statement
Call the Add method on the context's Courses DbSet property to add the course parameter to the context
Call the context's SaveChanges method to persist the changes to the database
}
}
public static void AddCourse(Course course)
{
using (var context = new Context())
{
context.Courses.Add(course);
context.SaveChanges();
}
}
public static void UpdateCourse(Course course)
{
// TODO
using (var context = new Context())
{
context.Courses.Attach(course)
.Entry("Modified");
context.SaveChanges();
}
}
}
}
5 Answers
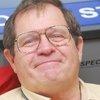
Joe Mackey
20,418 PointsMr Parker, Treehouse has some very odd behavior from time to time. The instructions are not part of my solution. Any other advice?
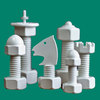
Steven Parker
231,268 PointsYour changes to AddCourse are correct. Good job.
But somehow, you copied the challenge instructions into the GetCourse method.
I'm sure the compiler was very confused!
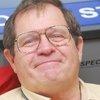
Joe Mackey
20,418 Pointsusing System.Collections.Generic; using System.Data.Entity; using System.Linq;
namespace Treehouse.CodeChallenges { public static class Repository { public static List<Course> GetCourses() { using (var context = new Context()) { return context.Courses .OrderBy(c => c.Teacher.LastName) .ThenBy(c => c.Teacher.FirstName) .ToList(); } }
public static List<Course> GetCoursesByTeacher(string lastName)
{
using (var context = new Context())
{
return context.Courses
.Where(c => c.Teacher.LastName == lastName)
.ToList();
}
}
public static Course GetCourse(int id)
{
using (var context = new Context())
{
return context.Courses
.Include(c => c.Teacher)
.SingleOrDefault(c => c.Id == id);
}
}
public static void AddCourse(Course course)
{
using (var context = new Context())
{
context.Courses.Add(course);
context.SaveChanges();
}
}
public static void UpdateCourse(Course course)
{
// TODO
using (var context = new Context())
{
context.Courses.Attach(course)
.Entry("Modified");
context.SaveChanges();
}
}
}
}
This does not pass for me. Throws this error:
Repository.cs(55,34): error CS1061: Type Treehouse.CodeChallenges.Course' does not contain a definition for
Entry' and no extension method Entry' of type
Treehouse.CodeChallenges.Course' could be found. Are you missing an assembly reference?
Course.cs(23,14): (Location of the symbol related to previous error)
Compilation failed: 1 error(s), 0 warnings
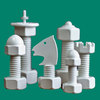
Steven Parker
231,268 PointsIf I copy and paste in the contents of your AddCourse method, it passes. But it looks like you also added some code for another method named "UpdateCourse". Is the button in the upper right going to the correct challenge?
In case you're working on the next challenge, I'll pass along the hints I gave to Amy Shah back in March when she happened to construct nearly the exact same code as you have here:
- like SaveChanges, Entry is a method of the context itself
- the Entry method returns a DbEntityEntry object that has a State property
- the State property can be set, but not using a string
- check the EntityState documentation for more info on possible values.
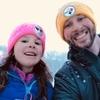
Dale Sublett
14,231 PointsHey Steven, I am getting this error...
(Repository.cs(55,32): error CS1061: Type System.Data.Entity.DbSet' does not contain a definition for
Entry' and no extension method Entry' of type
System.Data.Entity.DbSet' could be found. Are you missing an assembly reference?
/usr/local/lib/ruby/gems/2.3.0/gems/challenge_proctor-0.0.0/lib/challenge_proctor/runners/includes/mono/EntityFramework.dll (Location of the symbol related to previous error)
Compilation failed: 1 error(s), 0 warnings)
This is my code attempt.
public static void UpdateCourse(Course course)
{
using (var context = new Context())
{
context.Courses.Attach(course);
context.Courses.Entry(course).State = EntityState.Modified;
context.SaveChanges();
}
}
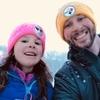
Dale Sublett
14,231 PointsNevermind. I just got it.
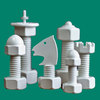
Steven Parker
231,268 PointsOK, but for future issues, always create a new question when you need help.
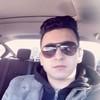
Oualid Jniyah
21,607 Pointsusing (var context = new Context()) { context.Courses.Attach(course); context.Entry(course).State = EntityState.Modified; context.SaveChanges(); } You passed "Modified as a Parameter while the question is to set the state of Entry to Modified! it took me a while to figure it out!
Steven Parker
231,268 PointsSteven Parker
231,268 PointsI'm looking above
at the code that was in the challenge when you selected "get help". At about line 36 the instructions seem to be added onto a line of code. That's the only problem, the code you added to AddCourse looks good and when pasted (by itself) into the challenge, it passes.