Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial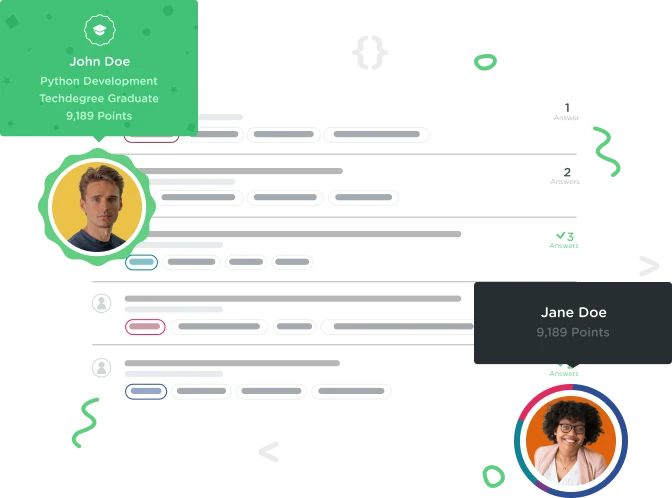

Amy Shah
25,337 PointsIn Repository.cs update the GetCourses method to sort the list of available courses by the teacher's last name and then
I need help because this is not compiling. I have reviewed that I need to orderby last name thenby first name. I think there is a syntax error
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
namespace Treehouse.CodeChallenges
{
public class Course
{
public Course()
{
Students = new List<CourseStudent>();
}
public int Id { get; set; }
public int TeacherId { get; set; }
[Required, StringLength(200)]
public string Title { get; set; }
public string Description { get; set; }
public int Length { get; set; }
public Teacher Teacher { get; set; }
public ICollection<CourseStudent> Students { get; set; }
}
}
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class Repository
{
public static List<Course> GetCourses()
{
using (var context = new Context())
{
return context.Courses.ToList()
.Where(c => c.Teacher.LastName == lastName)
.OrderBy(c => c.Teacher.LastName)
.ThenBy(c => c.Teacher.FirstName)
.ToList();
}
}
public static List<Course> GetCoursesByTeacher(string lastName)
{
using (var context = new Context())
{
return context.Courses
.Where(c => c.Teacher.LastName == lastName)
.ToList();
}
}
}
}
namespace Treehouse.CodeChallenges
{
public class Teacher
{
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
}
}
2 Answers
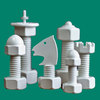
Steven Parker
231,269 PointsYou're pretty close, but:
- you should only call ToList() once, at the end of the LINQ expression
- the challenge did not ask you to filter the list
- there's no lastName parameter defined in this method to use in filtering anyway

Steven Scott
7,542 PointsThis passed for me.
using System.Collections.Generic; using System.Data.Entity; using System.Linq;
namespace Treehouse.CodeChallenges { public static class Repository { public static List<Course> GetCourses() { using (var context = new Context()) { return context.Courses.ToList(); } }
public static List<Course> GetCoursesByTeacher(string lastName)
{
return null; // TODO
}
}
}
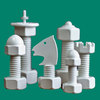
Steven Parker
231,269 PointsThat's not a valid solution. If the challenge accepted it, you have discovered a bug in the evaluation mechanism.