Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial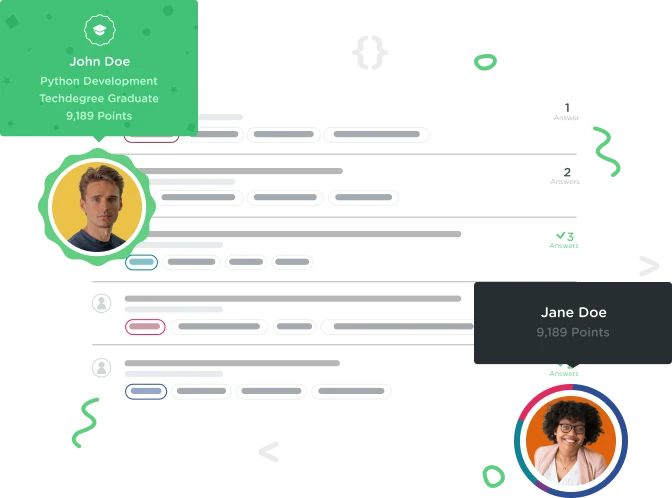
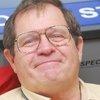
Joe Mackey
20,418 PointsIn Repository.cs update the GetCourses method to sort the list of available courses by the teacher's last name and then
I thought this was a slam dunk.
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
namespace Treehouse.CodeChallenges
{
public class Course
{
public Course()
{
Students = new List<CourseStudent>();
}
public int Id { get; set; }
public int TeacherId { get; set; }
[Required, StringLength(200)]
public string Title { get; set; }
public string Description { get; set; }
public int Length { get; set; }
public Teacher Teacher { get; set; }
public ICollection<CourseStudent> Students { get; set; }
}
}
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class Repository
{
public static List<Course> GetCourses()In Repository.cs update the GetCourses method to sort the list of available courses by the teacher's last name and then by their first name.
Remember, you can only make a single call to the OrderBy LINQ operator. To sort by additional properties, you can use the ThenBy operator.
{
using (var context = new Context())
{
return context.Courses.ToList();
.Where(c => c.Teacher.LastName == lastName)
// list of available courses by the teacher's last name
.OrderBy(c => c.Teacher.LastName)
// and then by their first name
.ThenBy(c => c.Teacher.FirstName)
.ToList();
}
}
public static List<Course> GetCoursesByTeacher(string lastName)
{
using (var context = new Context())
{
return context.Courses
.Where(c => c.Teacher.LastName == lastName)
.ToList();
}
}
}
}
namespace Treehouse.CodeChallenges
{
public class Teacher
{
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
}
}
1 Answer
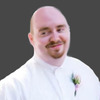
Rodger Voelkel
21,736 PointsI think you got yourself a little mixed up trying to copy the GetCoursesByTeacher method. You are also calling the ToList() method twice.
return context.Courses.OrderBy(c => c.Teacher.LastName).ThenBy(c => c.Teacher.FirstName).ToList();
i believe that is the correct code for this challenge. the ToList method should be the last call made and the where statement is not part of the challenge and is not needed.