Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial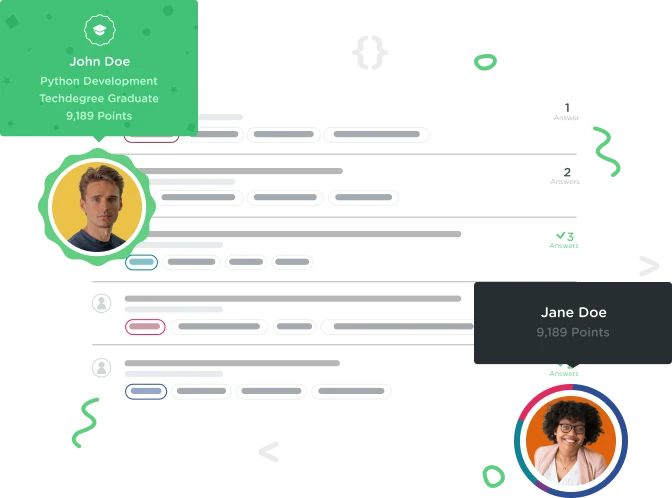
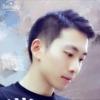
JUN MENG
10,249 Pointsin subclass, how we deal with the properties we didn't modified? Do we also need to initialize it?
in subclass, how we deal with the properties we didn't modified? Do we also need to initialize it? or the inherit method will do it under the hood.
4 Answers

Jorge Solana
6,064 PointsHey Jun!
In any Class, you need to initialize the properties you define. If you don't do it at the var/let declaration line, you get them through the init method. If you have a subclass with a new property initialized, you still have to initialize the pending properties from the superclass, but that's why you inherit the init method too, so you can use it to provide the value for them.
Here's a little example:
class Vehicle {
let wheels: Int
init(wheels: Int) {
self.wheels = wheels
}
}
class Car: Vehicle {
let seats: Int = 4
}
let myCar = Car(wheels: 4)
myCar.seats //this will return 4
myCar.wheels //this will return 4
myCar inherits wheels property and the Vehicle init method although it already has a seats property from his own. This time, in the subclass I don't need to modify that wheels property from the superclass since I'm already doing with my init method.
Hope this example is useful for you. Happy coding!

Stephen Emery
14,384 PointsI like the answer above. The short answer is we don't need to initialize the superclass's variables in the subclass, because they are being inherited as is when initializing the subclass.

akyya mayberry
3,668 PointsHmmm I thought it was said that we had to define the init() on a class no matter what?

Stephen Emery
14,384 PointsFor a regular and superclass, yes. Subclasses you don't need to define the init(). If you do, you will need to type "override" in front of the init() method inside the subclass.

nickmcgee
1,781 PointsI gathered this, too, from the videos -- my impression was that everything had to be initialized again from the sub-class, although this doesn't appear to be the case, which has led to really being confused as the examples don't follow his narration!
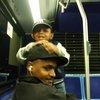
Xavier D
Courses Plus Student 5,840 PointsI agree with Stephen, you don't need to define the init() in a subclass unless you want the store properties that your subclass is inheriting from the superclass to be behave differently...for instance
class Person
{
let firstName: String
let lastName: String
init(firstName: String, lastName: String)
{
self.firstName = firstName
self.lastName = lastName
}
func fullName() -> String
{
return "\(firstName) \(lastName)"
}
}
class Mr: Person
{
override func fullName() -> String
{
return "Mr." + " " + lastName
}
}
Above is the superclass called Person, and a subclass called Mr. As you see, the superclass has an init method but the subclass does not. The subclass doesn't have an init method because I don't want to change anything about how the stored properties behave--I want firstName and lastName to function like how they function in the superclass. However, I do want change how the fullName method behaves....
let somePerson = Person(firstName: "Jorge", lastName: "Solana")
somePerson.fullName()
let someMister = Mr(firstName: "Jorge", lastName: "Solana")
someMister.fullName()
As you will see in Xcode, if you call the fullName method from both classes, you will notice that they both display the full name of someone differently. The subclass fullName method performs differently I had it override the superclass's fullName method and return something else