Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial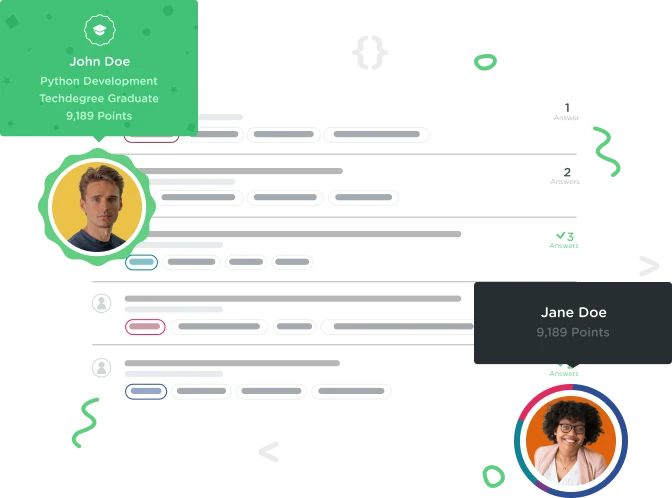

zhengyangdong
2,623 PointsIn Swift 2.0, shall I use as! or as?
The course was recorded when Swift 2.0 was not released yet. I noticed that in Swift 2.0 you have to choose between as! and as? for down casting. Which one shall I use?
init(index: Int) {
let musicLibrary = MusicLibrary().library
let playlistDictionary = musicLibrary[index]
title = playlistDictionary["title"] as! String!
description = playlistDictionary["description"] as! String!
let iconName = playlistDictionary["icon"] as! String!
icon = UIImage(named: iconName)
let largeIconName = playlistDictionary["largeIcon"] as! String!
largeIcon = UIImage(named: largeIconName)
artists += playlistDictionary["artists"] as! [String]
}
In this code for example, I tried using as? for each down casts but the compiler gives me an error:
While these two lines are perfectly okay: title = playlistDictionary["title"] as? String! description = playlistDictionary["description"] as? String!
These lines give me errors: let iconName = playlistDictionary["icon"] as? String! icon = UIImage(named: iconName) let largeIconName = playlistDictionary["largeIcon"] as? String! largeIcon = UIImage(named: largeIconName) artists += playlistDictionary["artists"] as? [String]
The compiler says that "Value of optional type String!? not unwrapped; did you mean to use ! or ?"
I really don't get the difference between as? and as!. As for the concept of down casting, I believe it is also unfamiliar for many people. I really hope that treehouse can make a separate course talking about these.
1 Answer
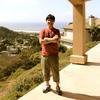
Richard Lu
20,185 PointsHey Zhengyang,
So the question, from what I understand, is when to use as!
and as?
. In order to find out which of the as
to use, you'll need to know what type of value you're assigning. I've provided some examples below.
as!
-
as!
is used when you're sure that the value is of that type, for example:
var aString: String = "Some string value" // This is a String for sure
var anotherString = aString as! String // Can be cast to a String for sure
as?
-
as?
is used to safely check the value type. If the value is not what it is, then the rest of that line is ignored. Here's an example
var x: String = "Zhengyang" // This is a String
var y = x as? Int // Can not be cast to an Int, therefore the value is nil
For the example for as?
, if we replaced it with as!
:
var x: String = "Zhengyang" // This is a String
var y = x as! Int // The as! in this case is stating I KNOW FOR SURE X IS AN INT, which really isn't and would cause the program to crash.
Let me know if there are any additional questions :)
- Rich