Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial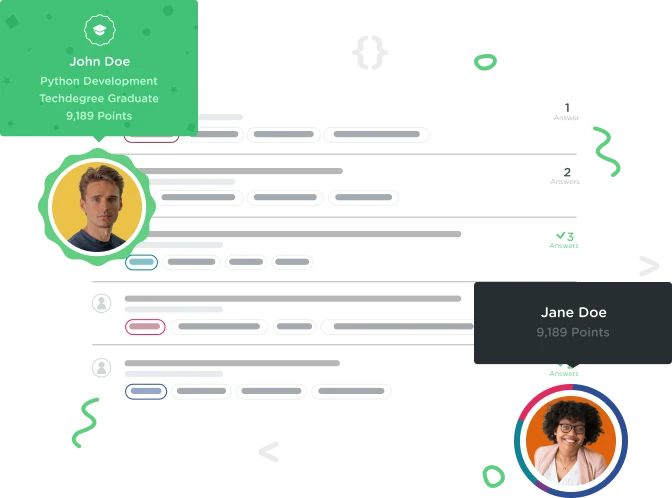

Siavash Gheifardi
12,510 PointsIn the callback function, log out the response's statusCode.
var http= require("http");
http.get("http://teamtreehouse.com/chalkers.json", function(response) {
//function properties go here
});
I really dont understand what it wants. Challenge one Task 4 of the Node.JS Basics.
4 Answers

Susan Rusie
10,671 PointsIf you type in the following code, you will pass the challenge. I hope this helps.
var http = require('http');
http.get("http://teamtreehouse.com/chalkers.json",function(response){
console.log(response.statusCode);
});

Aidan Lowson
10,379 PointsYou can also use an arrow function to get the same result
const https = require('https');
https.get("https://teamtreehouse.com/chalkers.json", response => {
console.log(response.statusCode);
});

Victoria Medley
17,085 PointsIt is simply asking you to log the status code for the response to the console. It can be accomplished using the following code:
var http= require("http");
http.get("http://teamtreehouse.com/chalkers.json", response => {
console.log(response.statusCode);
});
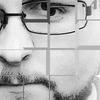
Jan Felix Trettow
19,833 PointsSo it just wants you to show that you know how to access the properties of the response object.
http's get method sends a GET request to the URL you gave as the first argument to the get method. As soon as the http.get method receives a response from the server (in this case from teamtreehouse.com), it calls the callback function you put in as the second argument AND passes in the response object (all the data the server send back in the response) as an argument for that callback function. By adding the response-parameter to your anonymous function in the previous step of the challenge you "catch" that response object and can now access it with the response variable (or whatever you called it). One of the properties of that object is .statusCode, which is a number that can tell you what happened (200 -> "I found the URL your were looking for and everything is ok", 404 -> "the server says it can't find that file", and many more).
The key thing here is the "catching". You just need to know that your anonymous callback function will be passed the response object. You can then (for this task) console.log() the statusCode-property of this response-object.