Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial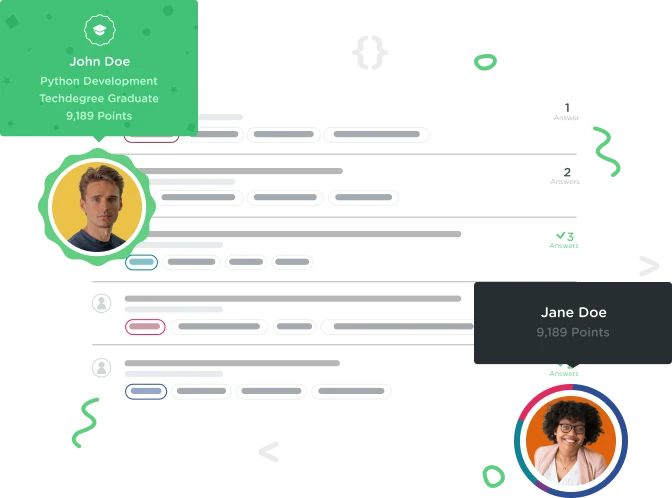

vincent batteast
51,094 PointsIn the ContactDao interface, add a findAll method that returns a List of Contact objects. Then, in ContactDaoImpl.java i
Bummer! 2 out of 2 tests failed. See preview for more details.
package com.teamtreehouse.contactmgr.dao;
import com.teamtreehouse.contactmgr.model.Contact;
import java.util.List;
public interface ContactDao {
List<Contact> findAll();
}
package com.teamtreehouse.contactmgr.dao;
import com.teamtreehouse.contactmgr.model.Contact;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class ContactDaoImpl implements ContactDao{
@Autowired
private SessionFactory sessionFactory;
@Override
@SuppressWarnings("unchecked")
public List<Contact> findAll(){
Session session = sessionFactory.openSession();
List<Contact> Contact = session.createCriteria(Contact.class).list();
session.close();
return null;
}
}
4 Answers
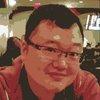
J Tan
11,847 PointsThe following code passed the challenge for me.
Interface
package com.teamtreehouse.contactmgr.dao;
import com.teamtreehouse.contactmgr.model.Contact;
import java.util.List;
public interface ContactDao {
List<Contact> findAll();
}
Impl class
package com.teamtreehouse.contactmgr.dao;
import com.teamtreehouse.contactmgr.model.Contact;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class ContactDaoImpl implements ContactDao {
@Override
@SuppressWarnings("unchecked")
public List<Contact> findAll() {
// Open a session
/* Session session = sessionFactory.openSession();
// DEPRECATED as of Hibernate 5.2.0
// List<Category> categories = session.createCriteria(Category.class).list();
// Create CriteriaBuilder
CriteriaBuilder builder = session.getCriteriaBuilder();
// Create CriteriaQuery
CriteriaQuery<Category> criteria = builder.createQuery(Category.class);
// Specify criteria root
criteria.from(Category.class);
// Execute query
List<Category> categories = session.createQuery(criteria).getResultList();
// Close session
session.close();*/
return null;
}
}

Seth Kroger
56,413 PointsThe main thing you still need to do is return the list of contacts you retrieve. You are currently just returning null. It's probably a good idea to rename the variable for that so it's not the same name as the class.

vincent batteast
51,094 Pointsit didn't work
package com.teamtreehouse.contactmgr.dao;
import com.teamtreehouse.contactmgr.model.Contact;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class ContactDaoImpl implements ContactDao{
@Autowired
private SessionFactory sessionFactory;
@Override
@SuppressWarnings("unchecked")
public List<Contact> findAll(){
Session session = sessionFactory.openSession();
List<Contact> Contact = session.createCriteria(Contact.class).list();
session.close();
return Contact;
}
}
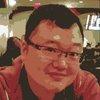
J Tan
11,847 PointsThe instruction states for now, it can return "null". So it should work.

vincent batteast
51,094 PointsI have been trying to work through this but it not working
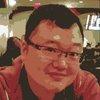
J Tan
11,847 PointsI think how I solved it was comment out everything in the method except for the return statement. I realized they were asking to create the method structure, not the individual statements in the method.

vincent batteast
51,094 Pointsstill now working
package com.teamtreehouse.contactmgr.dao;
import com.teamtreehouse.contactmgr.model.Contact;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class ContactDaoImpl implements ContactDao{
@Autowired
private SessionFactory sessionFactory;
@Override
@SuppressWarnings("unchecked")
public List<Contact> findAll(){
// Session session = sessionFactory.openSession();
// List<Contact> Contact = session.createCriteria(Contact.class).list();
// session.close();
return null;
}
}
vincent batteast
51,094 Pointsvincent batteast
51,094 PointsI don't see a different but it work form me too. ??
J Tan
11,847 PointsJ Tan
11,847 PointsWithout seeing your error output I could only make a guess. It could be the
@Autowired
annotation that I omitted.When I added the two lines of code for autowired I get the following compiler error:
It's also explained here: https://teamtreehouse.com/community/spring-hibernate-quiz-problem