Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial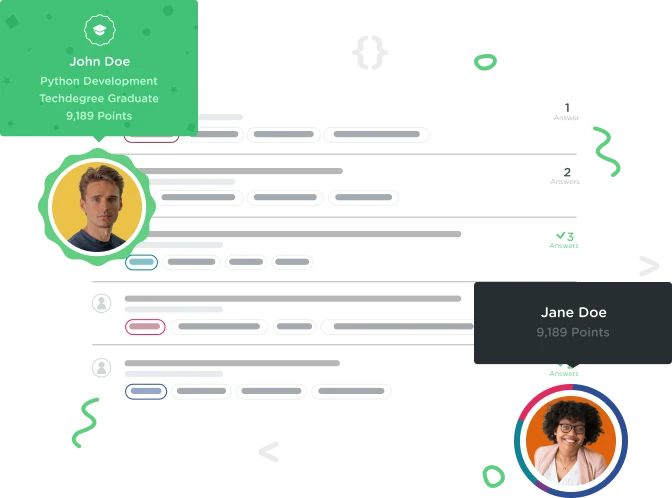

Shubham Agrawal
7,169 PointsIn the copyFile() method, why use a while loop?
In the copyFile() method, the write() method is writing "read" number of bytes to "buffer". If this step occurs as a single step, why use a while loop?
Code:
private static void copyFile(InputStream in, OutputStream out) {
byte[] buffer = new byte[1024];
int read;
while((read=in.read(buffer))!=-1){
out.write(buffer, 0, read);
}
}
1 Answer

David Petersheim
8,366 PointsA while loop is used because it may take more than one read to get all of the bytes transferred. This could be caused by one of two things.
Either the buffer is smaller than the file
If the buffer is smaller than the file, say a 10-byte buffer and a 40-byte file, then it will take at least 4 reads to get all 40 bytes from the file.
Or the read method doesn't read the whole file at once
The read method returns an integer that tells the caller how many bytes were read from the stream. If -1 is returned then the stream has ended.
The test in the while loop checks for -1. If -1 is returned, then the job is over. If another number is returned, then that number of bytes is written to the output stream.
The code is using a 1024-byte buffer. If
James O'Kelly
12,790 PointsJames O'Kelly
12,790 PointsIt is true that it may take more that one read, but it should increment the offset (which is currently always 0) in the output buffer by the amount of bytes read, because it currently would overwrite the buffer each time around the loop as it currently stands.