Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial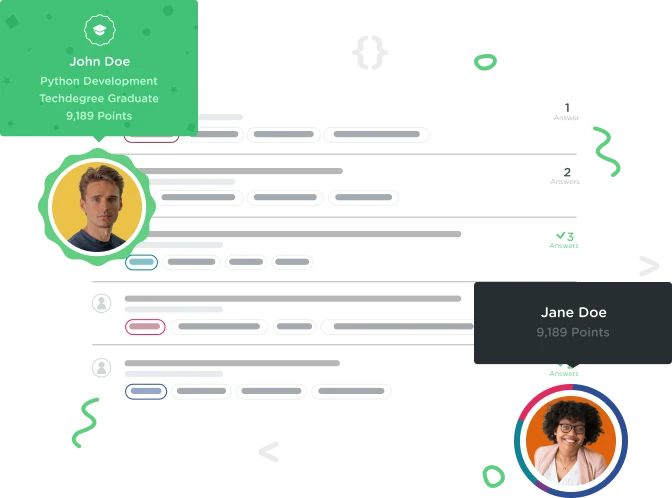

Wesley Lang
14,612 PointsIn the editor define a function named map with two generic type parameters, T and U. The function takes two arguments -
I do not know why my code is not working... I chose go as a var because I couldn't think of anything else
func map<T , U> (array : [T] , transformation : (T) -> U) -> [U]{
var go : [U] = []
for i in 0..<array.count {
go.append(array[i] * array[i])
}
return go
}
3 Answers
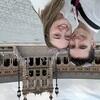
Grant Jason
10,713 PointsCouple of things to note here:
First, when defining the new array of U, you have to declare the new array instead of creating an array and assigning an empty array to it. Arrays are an Objective-C structure and can't be initialized with generic types. It requires a different method to declare it which I've put below.
var newArray = [U]()
Next, you don't have to specify the values the for loop is going to iterate over. You can just say "for (name) in (array)" and the for loop will automatically count the values in the array and stop execution once it reaches the last value.
From the looks of it, you're performing a transformation to your array without using the transformation parameter in the function declaration. All you have to do is pass in the transformation parameter into the append method you're calling on the new array in the loop and then pass in the value from the old array to the transformation function.
Once this is all done, return your new array and everything should pass. I've put my solution below for reference.
func map<T, U>(array: [T], transformation: (T) -> U) -> [U] {
var newArray = [U]()
for values in array {
newArray.append(transformation(values))
}
return newArray
}
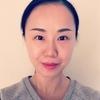
Yuqing Lu
7,032 Pointsfunc map<T, U>(array: [T], transformation: (T) -> U) -> [U] { var resultArray = U for item in array { resultArray.append(transformation(item)) } return resultArray }
it works for me
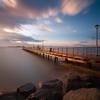
Qasa Lee
18,916 Pointsfunc map<T, U>(array: [T], transformation: (T) -> U) -> [U] {
var a:[U] = []
for item in array {
a.append(transformation(item))
}
return a
}
Wesley Lang
14,612 PointsWesley Lang
14,612 PointsThanks, this really helped.