Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial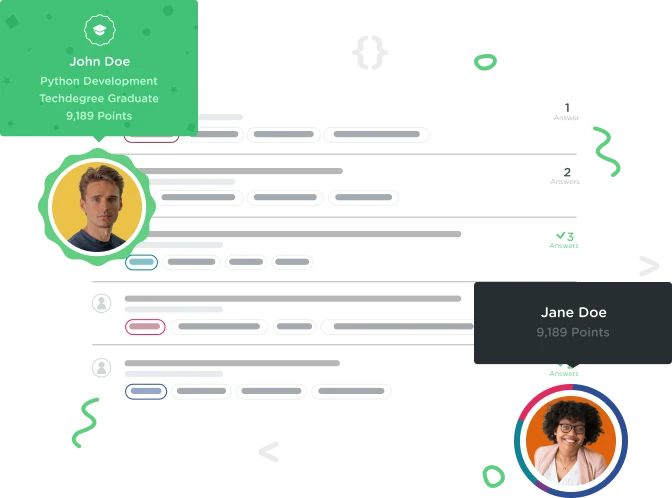

Junaid Shaikh
5,918 PointsIn the editor you've been provided with two classes - Point to represent a coordinate point and Machine. The machine has
how solve it
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
}
class Robot: Machine {
var location2: Point
var x1: Int
var y1: Int
override init() {
super.init(direction: String,x: x1,y: y1)
x1 = x
y1 = y
}
override func move(direction: String, x: x1, y: y1) {
switch direction {
case "Up":
++y1
case "Down":
--y1
case "Left":
--x1
case "Right":
--x1
default:
break
}
}
}
// Enter your code below
2 Answers
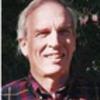
jcorum
71,830 PointsJunaid, good start, but as you saw there are some problems. First, you did too much work. You don't need the member variables in Robot, or the initializer. What variables are needed are inherited from Machine. And the default member-wise initializer works fine.
Second, in the overridden function you only need one parameter: direction.
Third, in the switch statement you need to reference the x and y variables of the Point object location, and increment them correctly. Increment should be in the form x++ or x--, not ++x or --x. With these changes you get:
class Robot: Machine {
override func move(direction: String) {
switch direction {
case "Up":
location.y++
case "Down":
location.y--
case "Left":
location.x--
case "Right":
location.x++
default:
break
}
}
}
Hope this helps.

Junaid Shaikh
5,918 PointsThank you