Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial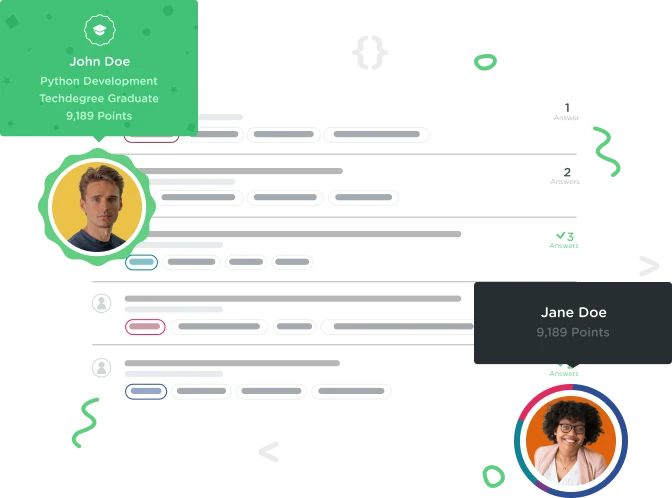

Piyawit Wipoosiri
Courses Plus Student 7,122 PointsIn the extra credit of "Parameters and Tuples", What is a syntax for a function that has Dictionary as parameter.
I can't figure out how to write the function that you give in the extra credit. Would you please show the correct answer or example.
2 Answers

Dan Chambers
6,057 PointsThis is what I came up with. It took me ages I must admit. I found it to be more of a syntax/documentation challenge than anything.
import UIKit
func nowPlaying (#song: Dictionary<String,String>) -> (title: String, artist: String, album: String) {
let title = song["Title"]
let artist = song["Artist"]
let album = song["Album"]
return (title: title!, artist: artist!, album: album!)
}
let song1 = [ "Title":"Singing in The Rain",
"Artist":"Frank Sinatra",
"Album":"Best of Frank"]
let currentSong = nowPlaying(song: song1)
println("The song that is currently playing is \(currentSong.title) by \(currentSong.artist) from the album \(currentSong.album).")

Stone Preston
42,016 Pointsthere are two styles of stating dictionary types.
the swift eBook section on collection types states:
The type of a Swift dictionary is written in full as
Dictionary<Key, Value>
, where Key is the type of value that can be used as a dictionary key, and Value is the type of value that the dictionary stores for those keys.
so we could create a function that takes a dictionary parameter with strings as its values and keys like so:
func someFunct(dict: Dictionary<String: String>) {
}
the eBook then states:
You can also write the type of a dictionary in shorthand form as
[Key: Value]
. Although the two forms are functionally identical, the shorthand form is preferred
so could create the same function using shorthand syntax for the parameter
func someFunct(dict: [String: String]) {
}

Piyawit Wipoosiri
Courses Plus Student 7,122 PointsThank you for your answer.... I try to create a function that way and there is no error. But I still try to call that function by passing a Dictionary Parameter in it, but always error. (I tried in Playground, Xcode) I also still find a sample syntax to use Dictionary Parameter which put in the function, e.g. println() but nothing happen...?
Thank you very much.
Kishan Solomon
1,135 PointsKishan Solomon
1,135 PointsWhat do the bangs(exclamation marks) do for the nowPlaying returns? i.e. title: title! instead of just title:title.
Robin Nilsson
3,783 PointsRobin Nilsson
3,783 PointsThe exclamation marks seem to be required (just using "title: title," produces an error in Xcode). The syntax is introduced for Optionals in the next video in the course. Does this mean that the individual values in a Tuple is actually counted as Optionals and need to be unwrapped just like Optionals do?
Austin Langley
5,392 PointsAustin Langley
5,392 PointsAnswer to:
What do the bangs(exclamation marks) do for the nowPlaying returns? i.e. title: title! instead of just title:title.
I had the same question as Kishan Solomon. I found the answer online:
The constants that are being created here will be automatically typed as optional by Swift because the keys may or may not exist in the dictionary. We use the bang to unwrap them.
I hope that helps.