Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial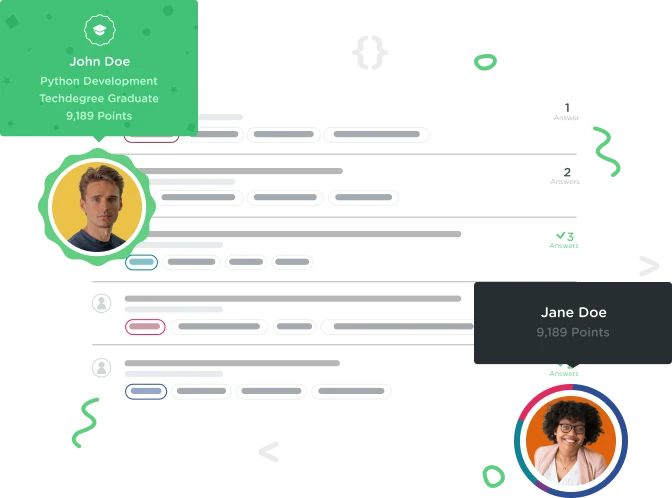

HAIDER AL RUSTEM
859 Pointsin the method: public String getAnswer(),
variable answer is inside the scope of this method, so how did java recognize it was the same as String answer at the very top? shouldnt String answer outside the scope of this method be static for the method to be able to return answer?
3 Answers
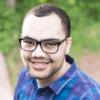
Philip Gales
15,193 PointsYou don't need the variable to be declared as static to be used in the class. But, the confusing part is there are 2 'answer' variables.
One (1) of the variables is declared at the top. This will be our class variable.
class Game {
public static final int MAX_MISSES = 7;
private String answer; //<---------------global variable answer
private String hits;
private String misses;
The other is a parameter in your method.
public Game(String answer) { //<-----local variable answer
Because the local variable answer is defined in the method signature, you are able to use it within the method. To differentiate between the two, you will have to refer to the global variable as 'this.answer'.
public Game(String answer) {
hits = "";
misses = "";
//the first answer is the global variable,
//the second answer is is from the method signature
this.answer = answer.toLowerCase();
}
You can rewrite and change the parameter, allowing you to drop the 'this' in reference to the global variable.
public Game(String newAnswer) {
hits = "";
misses = "";
//the first answer is the global variable,
//the second newAnswer is is from the method signature
answer = newAnswer.toLowerCase();
}
Now to your question specifically.
If there is only 1 answer, you do not have to tell the compiler which answer is which. It is by default the global answer.
If you did not tell the compiler in the previous example, it would have had to guess, and possibly break your game.
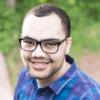
Philip Gales
15,193 PointsPlease post your code here so we can help. Without any code, no one will be able to assist/answer your question.

HAIDER AL RUSTEM
859 Points// the method in question is at the bottom
class Game {
public static final int MAX_MISSES = 7;
private String answer; private String hits; private String misses;
private char normalizedGuess (char letter){ if (! Character.isLetter(letter)){ throw new IllegalArgumentException (" a letter is required!"); } letter = Character.toLowerCase(letter);
if (misses.indexOf(letter) != -1 || hits.indexOf(letter)!= -1){
throw new IllegalArgumentException( letter+" Has been already tried! ");
}
return letter;
}
public boolean applyGuess ( String letters ) { if (letters.length() == 0 ){ throw new IllegalArgumentException (" must enter a vaild letter"); } return applyGuess(letters.charAt(0)); }
public boolean applyGuess (char letter){
letter = normalizedGuess(letter);
boolean isHit = answer.indexOf(letter) != -1;
if (isHit) { hits += letter;}
else {misses += letter;}
return isHit;
}
public String getProgress(){ String progress =""; for (char letter : answer.toCharArray()){ char display = '-'; if( hits.indexOf(letter) !=-1){ display = letter; } progress += display;} return progress; }
public int getRemainingTries(){ return MAX_MISSES - misses.length(); }
public Game(String answer){
hits =""; misses = ""; this.answer = answer.toLowerCase(); }
// this is the method, why answer is not initialized here or referred as this.answer ? how does java know it is the same answer above?
public String getAnswer(){ return answer; }
public boolean isWon(){
return getProgress().indexOf('-') == -1; }