Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial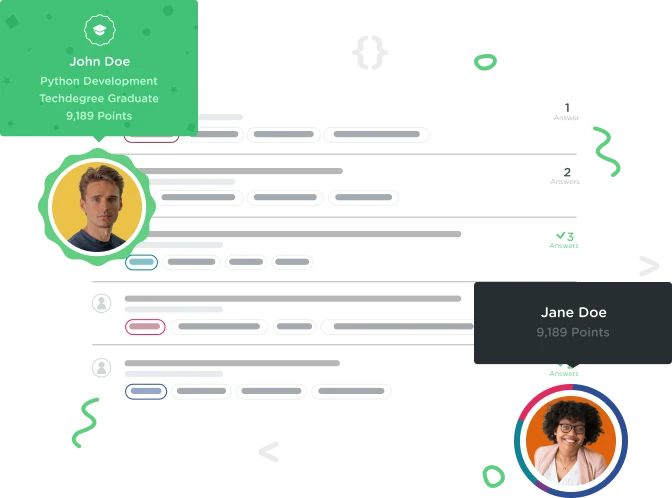

Patrick VanVorce
1,952 PointsIn the quiz following, it asks you to make a getTileCount member. Should it be of type int?
So I set my for member function as follows and I keep getting a compiler error:
public int getTileCount() { int count = 0; for ( char tile : mHand.toCharArray()) { if (hasTile) { count ++; } } return count; }
Shouldn't this suffice? What am I doing wrong?
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount() {
int count = 0;
for (char tile : mHand.toCharArray()) {
if (hasTile) {
count ++;
}
}
return count;
}
}
1 Answer
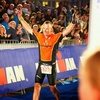
Steve Hunter
57,712 PointsHi Patrick,
The hasTile
method takes a parameter of type char
. So to use it in your if
statement would look like:
if (hasTile(tile))
However, the new method getTileCount
needs to receive a char
as a parameter too. It then returns the number of times that char appears in the mHand
string. You have the pssed-in parameter, so you then need to loop through the mHand
string (convert it to a char array, as you have done) - you then need to now if the parameter is equal to the current iteration element of the array. Just compare them directly.
Something like:
public int getTileCount(char tile){
int count = 0;
for (char letter : mHand.toCharArray()) {
if(letter == tile){
count++;
}
}
return count;
}
That's not hugely dissimilar to what you've written. But the key here is passing in the letter to be counted and then comparing that to the element of the array.
Make sense?
Steve.
Patrick VanVorce
1,952 PointsPatrick VanVorce
1,952 PointsThank you for your help, Steve.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNo problem!