Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial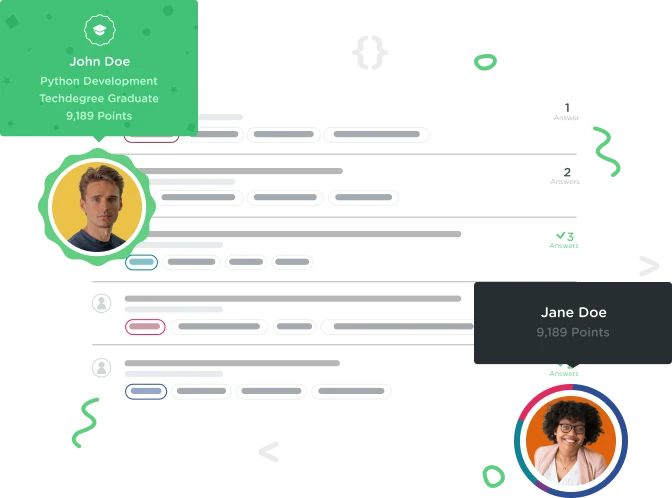
michaelabendroth
4,561 PointsIn the stage 4 delivering the MVP challenge, how do I check if the second letter in the field name is uppercase?
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) { // These things should be verified: // 1. Member fields must start with an 'm' if (! fieldName.startsWith("m")) { throw new IllegalArgumentException("Member fields must start with 'm'.\n"); } // 2. The second letter in the field name must be uppercased to ensure camel-casing
char fieldArray [] = fieldName.toCharArray();
if (! Character.isUpperCase(fieldArray[2])) {
throw new IllegalArgumentException("Fields must use camel-case.\n");
}
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
return fieldName;
}
}
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
if (! fieldName.startsWith("m")) {
throw new IllegalArgumentException("Member fields must start with 'm'.\n");
}
// 2. The second letter in the field name must be uppercased to ensure camel-casing
char fieldArray [] = fieldName.toCharArray();
if (! Character.isUpperCase(fieldArray[2])) {
throw new IllegalArgumentException("Fields must use camel-case.\n");
}
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
return fieldName;
}
}
1 Answer

Ken Alger
Treehouse TeacherMichael;
Remember that indexes in Java are 0 based, i.e. they start at zero (0). If we need to check the status of the second character in a string, that would reside at index position 1. A bit confusing at times, yes, but remember that we were age 0 for the first year of our lives too.
Post back if that clue didn't clear it up.
Happy coding,
Ken
michaelabendroth
4,561 Pointsmichaelabendroth
4,561 PointsYep, I had that part ok - I noticed that the test case that failed was in the format of m_field_name so I changed from using array position 1 to array position 2 to get the 2nd letter.
Thanks for replying back - I'll keep trying to work out where I mucked this one up. :)
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherMichael;
I got the code your posted above to pass the challenge just fine by changing:
Character.isUpperCase(fieldArray[2]))
which indexes the third character in the Array to
Character.isUpperCase(fieldArray[1]))
which indexes the second character in the Array.
Hope it helps.
Ken
michaelabendroth
4,561 Pointsmichaelabendroth
4,561 PointsThanks Ken! You are absolutely right - tried it again and it went right through. Appreciate it sir!