Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial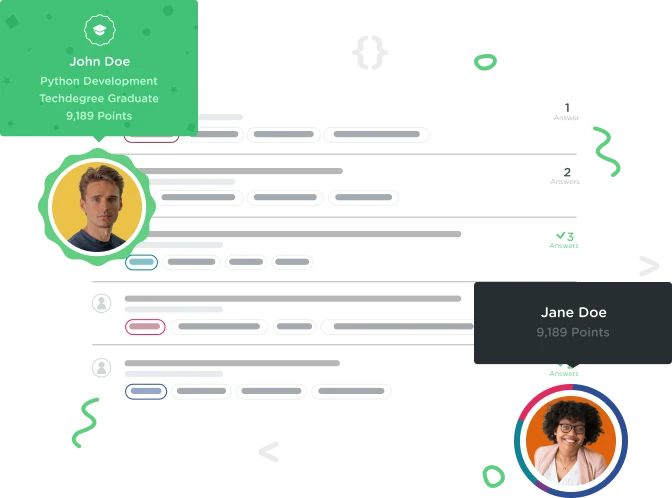
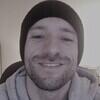
Paul Brubaker
14,290 PointsIn the word count challenge, is it bad practice to have my program raise an exception for each "new" word?
I am wondering if it is normal to have my program raise exceptions like this for each new word that is not found in the list as a normal part of the programs operation. That is, is it a bad habit to use exception raising in this way? Should I be doing this differently, or does it not really matter?
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
word_count_dict = {}
for word in string.lower().split():
try:
word_count_dict[word] += 1
except KeyError:
word_count_dict[word] = 1
return word_count_dict
1 Answer
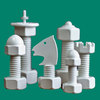
Steven Parker
229,771 PointsI'm not sure if this would constitute "bad practice", but rather than causing and catching errors you could easily perform a test to determine which action to take:
if word in word_count_dict:
word_count_dict[word] += 1
else:
word_count_dict[word] = 1
Paul Brubaker
14,290 PointsPaul Brubaker
14,290 PointsThat seems like a better may to do it. Thanks Steven! I am so new to this, that I'm sure the solutions I come up with will be "unnecessarily creative" at times and I understand that is something to be avoided in python.