Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial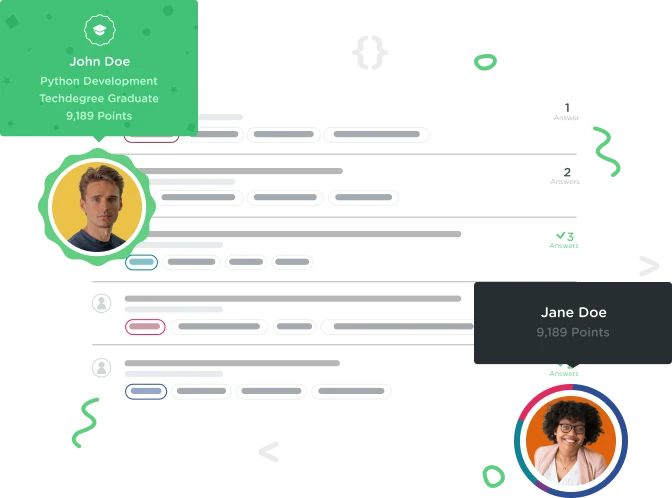
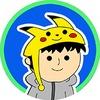
smatt
2,115 PointsIn this instance couldn't we just exempt the else statement? Why is it necessary?
while (keepGoing) {
//Prompt the user for minutes.
Console.Write("Enter how many minutes you exercised or type \"quit\" to exit: ");
string entry = Console.ReadLine();
if (entry == "quit")
{
keepGoing = false;
}
int minutes = int.Parse(entry);
// Add Minutes to total.
runningTotal = runningTotal + minutes;
// Display total minutes on screen
Console.WriteLine("You've excercised " + runningTotal + " minutes");
// Repeat until over.
}
Console.WriteLine("Application Closing.");
Surely with the code above, in this instance when the user enters "quit" then it would then just skip through the rest of the code all the way down to the Console.Writeline instead of attempting to run the int minutes = int.Parse(entry); etcetc. Essentially the same as if we did the code below.
using System;
namespace Treehouse.FitnessFrog { class Program { static void Main() {
int runningTotal = 0;
bool keepGoing = true;
while (keepGoing)
{
//Prompt the user for minutes.
Console.Write("Enter how many minutes you exercised or type \"quit\" to exit: ");
string entry = Console.ReadLine();
if (entry == "quit")
{
keepGoing = false;
}
else
{
int minutes = int.Parse(entry);
// Add Minutes to total.
runningTotal = runningTotal + minutes;
// Display total minutes on screen
Console.WriteLine("You've excercised " + runningTotal + " minutes");
// Repeat until over.
}
}
Console.WriteLine("Application Closing.");
}
} }
Why is it necessary for us to put the else loop in? Why is this a rule.
2 Answers

Emmanuel C
10,636 PointsIn the first example, without the else, the rest of the code would still run, even if they enter quit. That keepGoing bool wouldnt be evaluated until the end of the loop when it checks the while condition and decided if it should run again.
That program should actually crash if the user enters quit, since all the if statement does is set keepGoing to false, but doesnt break out of the loop, the int.Parse would still run, and crash trying to parse "quit" into a number.
In order to not have an else statement, you can use the keyword break in that if statement, which will make the program break out of the loop, like so...
while (keepGoing) {
//Prompt the user for minutes.
Console.Write("Enter how many minutes you exercised or type \"quit\" to exit: ");
string entry = Console.ReadLine();
if (entry == "quit")
{
keepGoing = false;
break;
}
int minutes = int.Parse(entry);
// Add Minutes to total.
runningTotal = runningTotal + minutes;
// Display total minutes on screen
Console.WriteLine("You've excercised " + runningTotal + " minutes");
// Repeat until over.
}
Console.WriteLine("Application Closing.");
Then you technically wouldnt need that bool keepGoing as a flag, since the break keyword will decide whether the loop ends or not.
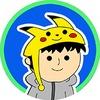
smatt
2,115 PointsThanks for the help :)
smatt
2,115 Pointssmatt
2,115 PointsI see. So in these tutorials obviously we haven't learnt about break yet. So I'm kind of jumping the gun? And I just tested the first one and noticed it was crashing as a matter of fact. Should have tested it without copying haha. But basically long story cut short, you always either need an else, or a break?
Emmanuel C
10,636 PointsEmmanuel C
10,636 PointsIt does depend on the situation, for this one, the else was needed, as the user entering "quit" is suppose to end the loop. So IF the user entered a number then do appropriate calculations, but(else) if the user entered "quit" than set the keepGoing flag to false and dont do the calculation. This is very common in programming, where you would have to do different things depending on what the user enters. If statements are a great way to decide what to do depending on what they entered. If the user enter this, then do this, else if the user enters that do something else and so on.