Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial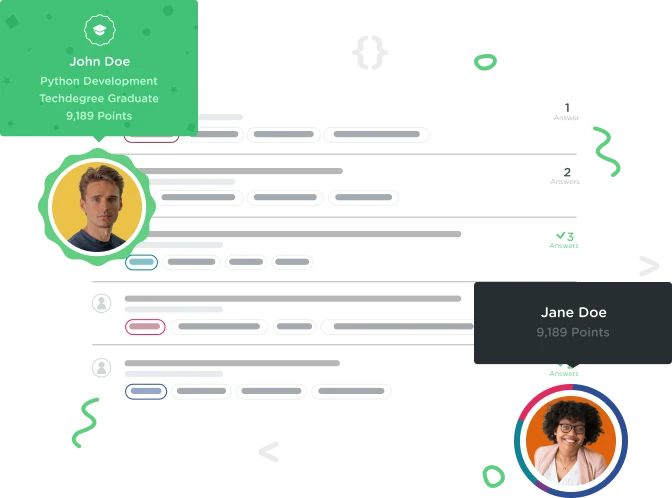

Radu - Adrian Buha
Courses Plus Student 5,535 PointsIn Using Dictionaries, I have a question for the second challenge.
Hello,
Apparently, I don't seem to get the value from the Dictionary. What am I doing wrong?
Many thanks in advance!
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class LexicalAnalysis
{
public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
int count = 0;
if(WordCount.ContainsKey(word))
{
WordCount[word] = WordCount[word] + 1;
}
else if(!(WordCount.ContainsKey(word)))
{
WordCount.Add(word,count+1);
}
}
public Dictionary<string, int> WordsWithCountGreaterThan (int counter){
Dictionary<string, int> WordsWithGreaterCounter = new Dictionary<string,int>();
foreach(KeyValuePair<string,int> searchedWord in WordCount){
if(WordCount[searchedWord.Value] > counter){
WordsWithGreaterCounter.Add(searchedWord.Key,searchedWord.Value);
}
}
return WordsWithGreaterCounter;
}
}
}
2 Answers
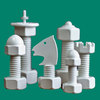
Steven Parker
230,274 PointsYou're so close! But when you check the count of each word you index the dictionary using the value ("WordCount[searchedWord.Value]
") but dictionaries are indexed using keys. And while changing "Value" to Key" will allow the code to pass the challenge, there's an even better solution. Since you already have the value, just use it directly in the comparison instead of using the key to look the value up again in the dictionary.

Radu - Adrian Buha
Courses Plus Student 5,535 PointsTHANK. YOU! :D It worked! Wuhuu!