Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial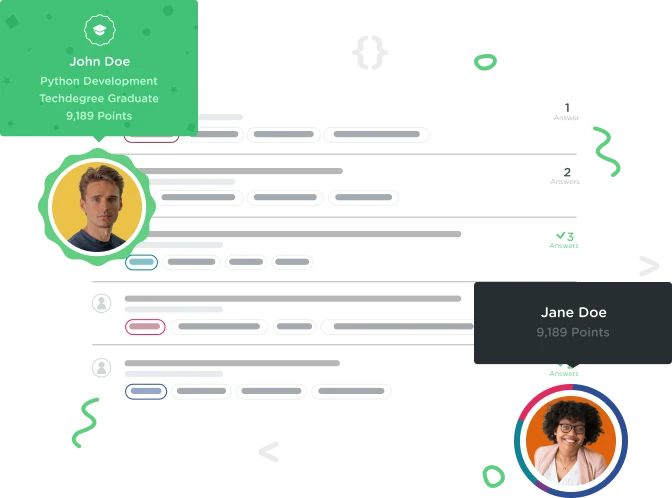
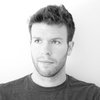
Matthew Rigdon
8,223 PointsInaccurate error regarding "double" type and "+=" operator?
I've seem to have written completely valid code (tested via REPL), but the challenge won't accept it. My current code is:
The error I'm receiving is: FrogStats.cs(12,17): error CS0019: Operator +=' cannot be applied to operands of type
double' and `Treehouse.CodeChallenges.Frog' Compilation failed: 1 error(s), 0 warnings
On https://repl.it/languages/csharp I've created a similar scenario and the code runes fine and doesn't produce the above error. Can someone explain why my current code isn't valid and what I can do to correct it?
Thanks!
From REPL.IT
using System;
class MainClass {
public static void Main (string[] args)
{
int[] numbers = new int[]{1,2,3,4};
double abc = FrogStats.GetAverageTongueLength(numbers);
Console.WriteLine(abc);
}
}
class FrogStats
{
public static double GetAverageTongueLength(int[] frogs)
{
double averageLength = 0;
for (int index = 0; index < frogs.Length; index++)
{
averageLength += frogs[index];
}
return averageLength/frogs.Length;
}
}
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
double averageLength = 0;
for (int index = 0; index < frogs.Length; index++)
{
averageLength += frogs[index];
}
return averageLength/frogs.Length;
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
3 Answers

andren
28,558 PointsTake a close look at the declaration of the GetAverageTongueLength
method from the workspace:
public static double GetAverageTongueLength(Frog[] frogs)
And then contrast it with the declaration from your repl code:
public static double GetAverageTongueLength(int[] frogs)
Can you notice a difference between them? Well there is a pretty big one actually, they take in completely different objects as parameters.
In the challenge you are not receiving an array of ints, but an array of Frog
objects. A Frog
is not a number, which is why you can't add it to a number using the +=
operator.
If you look at the Frog
class (which is available as a separate file in the challenge workspace) then you'll see that it has a property called TongueLength
. That is where the tongue length of the Frog
object is stored, and is what you have to access for your code to work.
To access this property on the Frog
object you simply need to add .TongueLength
like this:
for (int index = 0; index < frogs.Length; index++)
{
averageLength += frogs[index].TongueLength;
}
That is all you have to do to complete this challenge.
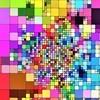
james south
Front End Web Development Techdegree Graduate 33,271 Pointsit looks like you are trying to add non-double types to your double variable. the method takes an array of Frogs. do you have a getter method perhaps that returns the length of a Frog's tongue? that could return a double, then be added to your double variable. you might also try expanding the += operator to var = var + (new value), instead of var+=(new value).
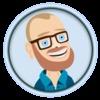
Justin Crawford
16,736 PointsHow would you do this for a "foreach" loop?
Matthew Rigdon
8,223 PointsMatthew Rigdon
8,223 Pointsandren Great explanation and that makes total sense! I appreciate the help.