Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial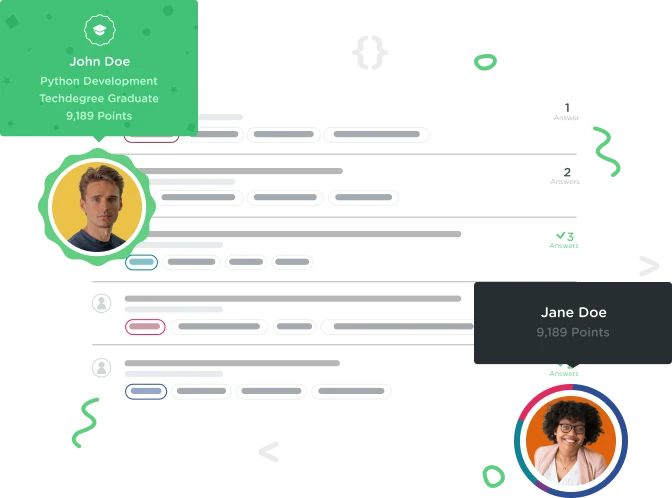
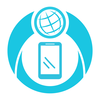
Joseph Cho
5,544 PointsInclude image & coordinates from external array
I have an external array that holds more individual arrays that represent a question, it's multiple choices, and the correct answer (if it's a multiple choice "mc" question) or if the question is labeled "diagram", the subarray holds a question, the directory to the image the question uses and the coordinates of the image map.
The external array is called "questions" that looks like this:
var questions = [
["mc",
"Question 1",
"Choice A",
"Choice B",
"Choice C",
"Choice D",
"B"],
["mc",
"Question 2",
"Choice A",
"Choice B",
"Choice C",
"Choice D",
"D"],
["mc",
"Question 3",
"Choice A",
"Choice B",
"Choice C",
"Choice D",
"B"],
["diagram",
"Question 4",
"img/facesmall.png",
[false, false, false, false, false, false],
[false, true, false, false, false, false],
"424,33, 460,10, 518,7, 534,10, 554,18, 570,37, 578,56, 581,79, 582,96, 584,124, 585,144, 584,166, 580,185, 576,210, 573,232, 570,256, 566,278, 566,301, 570,325, 578,343, 586,362, 598,376, 608,392, 616,410, 622,427, 624,451, 621,472, 614,493, 609,513, 605,538, 605,550, 611,563, 612,566, 604,559, 598,544, 595,529, 589,514, 584,503, 573,486, 565,471, 555,456, 543,445, 528,430, 513,419, 492,404, 477,396, 463,382, 459,378, 456,364, 456,351, 459,341, 462,330, 465,314, 462,301, 466,292, 467,283, 471,268, 473,251, 475,228, 475,211, 475,191, 474,172, 471,156, 468,142, 462,132, 462,122, 462,113, 464,102, 464,92, 459,80, 452,69, 448,69, 441,57, 424,35"]
];
and I am trying to use it in my javascript like so:
test.innerHTML += "<img src ='questions[pos][2]' class = 'map' usemap = '#sections'></img>";
pos is just a counter keeping track of what sub array (what question) we're currently dealing with.
I keep getting the error message that the image file can't be found. Any tips?
2 Answers
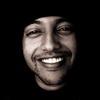
miikis
44,957 PointsHey Joseph,
I think the problem is that pos
variable that you're calling a "counter." Can't say for sure without seeing the rest of the code but, unless you have a loop in there somewhere ... pos
is just a random undefined variable.
If this line...
test.innerHTML += "<img src ='questions[pos][2]' class = 'map' usemap = '#sections'></img>";
...is just being used as a preliminary test (you wanna hard-code the array values), then it should look like this...
var test = document.getElementById("test"); //assuming you have some element with the id of "test" in your html
test.innerHTML += '<img src="' + questions[3][2] + '">'; //Previously you had the array as a string for some reason...
Assuming everything that Sara Hardy said to do is done, the above should work.
One more thing, at questions[3][5]
, you have this giant string... and the formatting suggests the values are supposed to be of the data type Number. If this is the case, those values need to be inside an array of their own... and don't use commas as a digit separator... or as a decimal point... use periods.

Sara Hardy
8,650 PointsThe first step would be to verify that you are getting the value you expect for the image. In this case "img/facesmall.png".
The next step would be to check that the path is correct. In JavaScript, paths are relative to the page, not to the javascript file itself. So for your path "img/facesmall.png", the image is expected to be in a folder called "img" that is within the same directory as the page you are viewing.
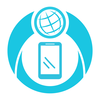
Joseph Cho
5,544 PointsI printed out what questions[pos][2] is to the console and it prints "img/facesmall.png" so I know I'm getting the value that I want.
I also know the path is correct because if I just hard code in "img/facesmall.png" for the src instead of "questions[pos][2]" then the image shows up fine... Any more suggestions? Thanks for the reply
Joseph Cho
5,544 PointsJoseph Cho
5,544 Pointspos is a counter that is being incremented appropriately. I confirmed this by printing to the console and questions[pos][2] prints the same thing as questions[3][2]. I refrained from copying all my code because there is a lot and I didn't this post to turn into just a giant clustermess, sorry haha. Anyway, changing the src to:
<img src =" + questions[pos][2] + " class = 'map' usemap = '#sections'></img>;
worked! The image now successfully shows up thank you.
Now I have an additional question. That giant string are a series of x1,y1, x2,y2, ... style coordinates for an image map. Currently I am trying to incorporate them into the javascript like so:
test.innerHTML += "<map class='tabs' name='sections'> <area id='ponytail' shape='poly' alt='' onclick='toggleSelection(5)' coords=" + questions[pos][5] + " href='#map2-info'/>
But after the image loads, I still can't click where the section of the image map is... any more pointers?
miikis
44,957 Pointsmiikis
44,957 PointsWell, other than the
img
tag being a self-closing tag (no end tag necessary), I can't tell what could be going wrong without seeing the rest of the code. Just copy it and post it man. The syntax highlighting on the Treehouse forum is actually kinda sick.