Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial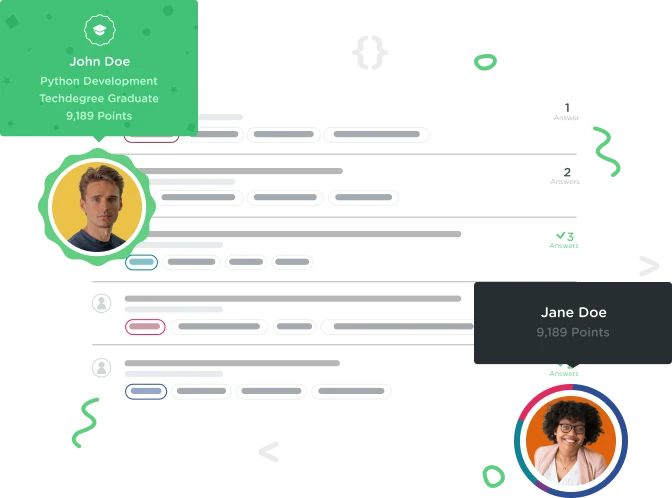

Angelina Prodromides
8,566 PointsIncluding Enumerable on class instead of using built in enumerable methods on the players array
In this video we included enumerable on the Game class so that we could say:
puts game1.any?{|player| player.score > 80}
So from what I'm understanding we are using the enumerable method of any? directly on an instance of our custom Game class and that's why we have to "include" it.
My question is why don't we just use the any? method directly on the players array since the array class already comes with this method? I tested this out (after deleting the line "include Enumerable" from the Game class) and it worked the same:
puts game1.players.any?{|player| player.score > 80}
Here is the full example given to us from the video if anyone needs to reference it:
class Player
include Comparable
attr_accessor :name, :score
def <=>(other_player)
score <=> other_player.score
end
def initialize(name, score)
@name, @score = name, score
end
end
class Game
include Enumerable
attr_accessor :players
def each(&block)
players.each(&block)
end
def initialize
@players = []
end
def add_player(player)
players.push(player)
end
def score
score = 0
players.each do |player|
score += player.score
end
score
end
end
player1 = Player.new("Jason", 100)
player2 = Player.new("Kenneth", 80)
player3 = Player.new("Nick", 95)
player4 = Player.new("Craig", 20)
game1 = Game.new
game1.add_player(player1)
game1.add_player(player2)
game2 = Game.new
game2.add_player(player3)
game2.add_player(player4)
puts game1.inspect
puts game1.any?{|player| player.score > 80}
players = game1.find{|player| player.score > 80}
puts "Players with a score > 80", players.inspect
1 Answer
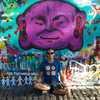
Andrew Stelmach
12,583 PointsYep, you're right. It works exactly the same. Well spotted - if this was a project, your version would arguably be better code (less is more).
In this case, I'm guessing Jason was just demonstrating what you can do if you really do need to use an Enumerable method.
Andrew Stelmach
12,583 PointsAndrew Stelmach
12,583 PointsI think an interesting question is, 'how does
game1.players
return an array of players? How doesattr_accessor
do this?'