Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial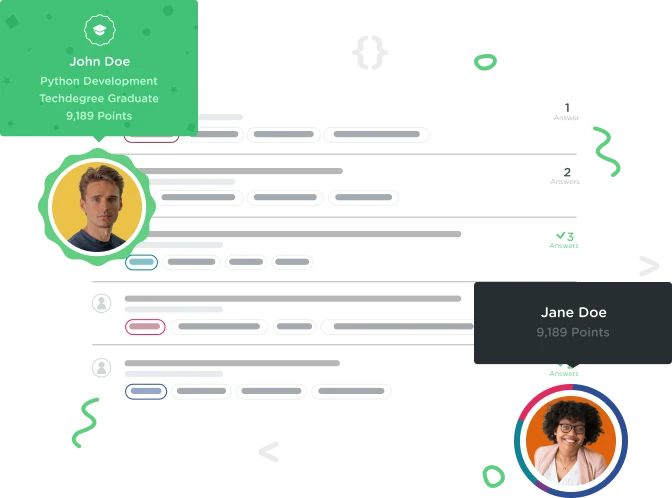
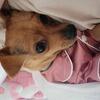
shiny peanut
5,113 PointsIndex of a Char in a String/Scrabble
Am I doing more than the problem asks for? I can't figure out why this isn't working.
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
private String hits;
private String misses;
public ScrabblePlayer() {
tiles = "";
hits = "";
misses = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
boolean hasIt = tiles.indexOf(char tile) != -1;
if (hasIt) {
hits += tiles;
return true;
} else {
misses += tiles;
return false;
}
}
1 Answer

andren
28,558 PointsYou are indeed doing more than what is asked for, though there is also a syntax error on top of that.
The method is named hasTile
and its only job is to answer that question, it is not supposed to do anything else. In your solution you have added code to it that categorizes the passed in tile into hits
and misses
strings, which you were not actually asked to do.
As for the syntax error that is found in this line:
boolean hasIt = tiles.indexOf(char tile) != -1;
You are only supposed to specify the type of a variable when you define it, not when you use an existing variable. Since tile
is an existing variable you should not include char
in front of it.
On top of those two issues it is also worth pointing out that you don't need to use an if
statement in this method, and the challenge checker will complain if you do. Since the hasIt
variable contains the Boolean value you want to return you can just return it directly without using it in a conditional like if
. Like this:
public boolean hasTile(char tile) {
boolean hasIt = tiles.indexOf(tile) != -1;
return hasIt; // Return Boolean stored in hasIt directly
}
Or even more succinctly like this:
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1; // Return the Boolean the expression results in
}
Since the expression is only used once storing it in a variable is not really necessary, but either solution will be accepted.