Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial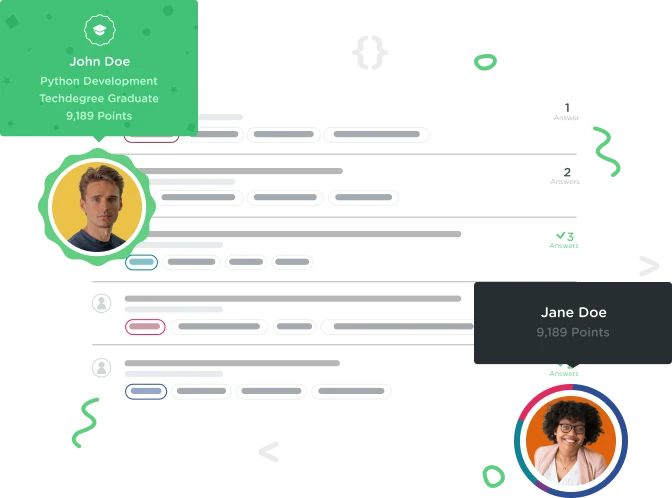
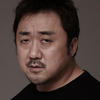
chanjong kim
2,315 Pointsindex out of range
iam returning the list of integers {1,2,4,8,16} and i got index out of range error. I think index should start from 0 so that it can represent 1 as 2powerd by 0. what is the wrong then in my code??
using System;
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class MathHelpers
{
public static List<int> GetPowersOf2(int number)
{
List<int> list = new List<int>();
for(int i =0; i<=number; i++)
{
list[i] = (int) Math.Pow(2,i) ;
}
return list;
}
}
}
1 Answer
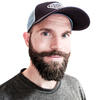
Daniel Breen
14,943 PointsYour list doesn't know what size it is.
Remember that a List is just a fancy container for an array.
try list.Add((int) Math.Pow(2,i));
instead of list[i] = (int) Math.Pow(2,i);
The .Add()
will resize the array for you and prevent IndexOutOfRangeException
Daniel Breen
14,943 PointsDaniel Breen
14,943 PointsI answered below, but your question made me wonder about List Capacity Initialization as well. My first thought was that you could initialize the List capacity...
List<int> list = new list<int>(number + 1)
...then do as you did...
list[i] = (int) Math.Pow(2,i) ;
However, I found from this stack overflow answer that it doesn't quite work like that.
https://stackoverflow.com/a/15843509/6843314
"There is List<T> constructor which takes an int as a parameter for initiall list capacity, but it does not actually creates that number of elements within a list, so this will throw ArgumentOutOfRangeException... "
So my answer below is the simple solution, but there's useful info in that stack overflow answer.