Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial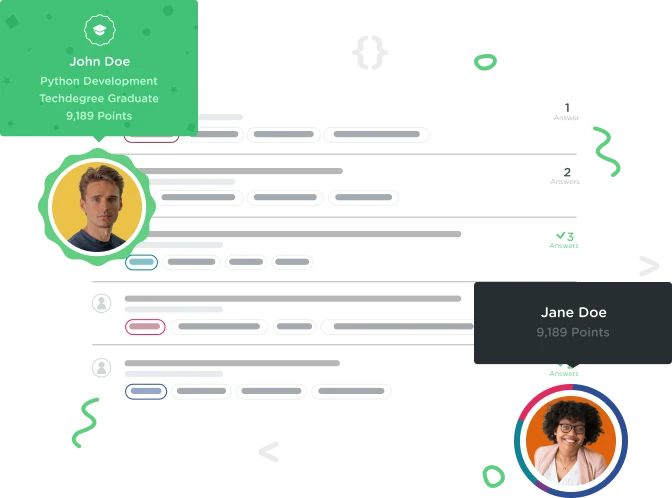
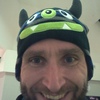
William LaMorie
11,769 PointsIndex searching vs conditionals?
I've got a silly question. I don't like how big stretches of conditionals look in code, and I think switches are ugly. It occurs to me that you could check the user input for the get_weapon with a lot less keystrokes and a lot less conditionals with something like:
def get_weapon(self):
weapon_choice = input("Weapon ([S]word, [A]xe, [B]ow): ").lower()
choices = {'a': 'axe', 'b': 'bow', 's': 'sword'}
if weapon_choice in choices:
return choices[weapon_choice]
else.....
dictionary look up is O[1] so it should run on the same sort of speed as a conditional checking a string, and it's a lot flatter... is there any reason that with any reasonable sized dictionary this wouldn't be a better solution?
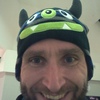
William LaMorie
11,769 PointsHi Ron,
Thanks for the reply! But actually, my code run as intended the way it is.
When you use in on a dictionary in python it's just going to look at the keys. if you try something simple like...
tst = {0:1}
print(0 in tst)
print(1 in tst)
..you'll see what I'm saying... Which is pretty awesome.
So, I'm not asking if the code works, I'm just wondering given that the performance is pretty much identical, and seeing that we don't even care about performance in something like this.. it's very explicit, it's much less nested, easier to expand out, I'd say it's much in line with PEP8 and just nice code...
but it seems like whenever I assume 'foo' is a better way to do something then 'bar' someone comes along and tells me I'm wrong... so I'm just asking if anyone can give me a good reason why we wouldn't want to use the dict rather than a whole slopload of conditionals.
-Will

Ron Fisher
5,752 PointsWill,
You are correct in the way your code works. I personally don't see a reason this is not good code. It is a little ambiguous looking to the unassuming.
I have always written code with the "if item in dictionary.keys()" method however, I like it and will use this method.
Ron
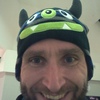
William LaMorie
11,769 PointsRon,
I'm pretty sure that dictionary.keys() method gives you a lot of speed if you're looking at traversing a very large static dictionary. If I recall I got some pretty good time reduction using something like node_names_bar = set([foo_nodes.keys()]) for another course I took on Python at some point, it's a good method.
1 Answer
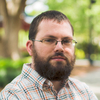
Kenneth Love
Treehouse Guest TeacherYeah, that's a fine way of doing it, too. You'll see people doing switches in Python with dictionaries a lot.
Ron Fisher
5,752 PointsRon Fisher
5,752 PointsWilliam,
I believe the speed isn't really a factor unless you have hundreds of thousands of choices. You can search on the web for "python dictionary internal implementation" and read more about how they work internally.
I believe you need one change to your code to make this a plausible solution.
if weapon_choice in choices.keys(): return choic........