Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial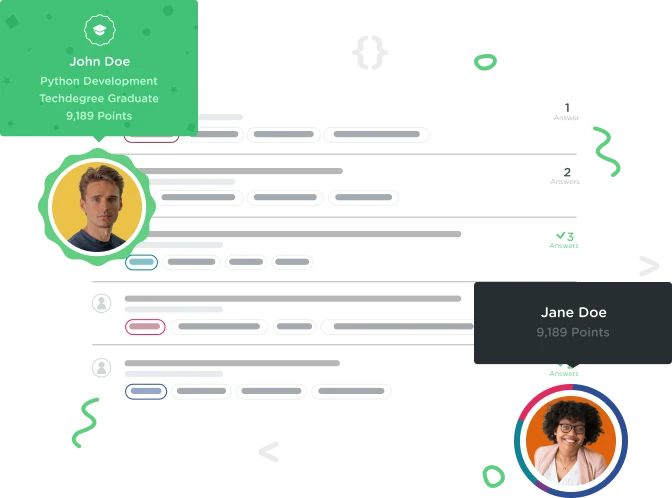

Tizmiz Tizzer
1,040 PointsIndex was outside the bounds of the array
I'm not sure what I'm doing wrong here. I just keep getting the same error.
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
int amount = 0;
double addFrog = 0.0;
for(int i = 0; i < frogs[i].TongueLength; i++)
{
addFrog = addFrog + frogs[i].TongueLength;
amount++;
}
return addFrog/amount;
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
1 Answer

andren
28,558 PointsThe issue is the run condition for your for loop: i < frogs[i].TongueLength
you are saying you want the loop an to run an equal number of times as the tongue length of the first frog. Which doesn't really make much sense. And will lead the loop to run far more times than it should, which results in i
being far higher than the amount of objects there actually are in the frogs
array.
In reality you want the loop to run once for each frog in the frogs
array. Arrays have a property called Length
which will tell you how many objects are within it. That is the property you should be using as the run condition.
Also your amount
variable is somewhat pointless since it ends up also just being a counter for how many objects there are in the array, which the Length
property already provides you.
If you use frogs.Length
in the run condition of the for
loop and remove your amount
variable and replace it with frogs.Length
like this:
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
double addFrog = 0.0;
for(int i = 0; i < frogs.Length; i++)
{
addFrog = addFrog + frogs[i].TongueLength;
}
return addFrog/frogs.Length;
}
}
}
Then your code will work.
Tizmiz Tizzer
1,040 PointsTizmiz Tizzer
1,040 PointsThanks, that helped. I might have been doing frogs.length at first and ended up trying frogs[i].TongueLength. Which didn't make sense to me either since as you said it'll be equal. I was going off another question asked on this video. Thank you for the help though! Just gotta watch out for capitalization next time.