Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial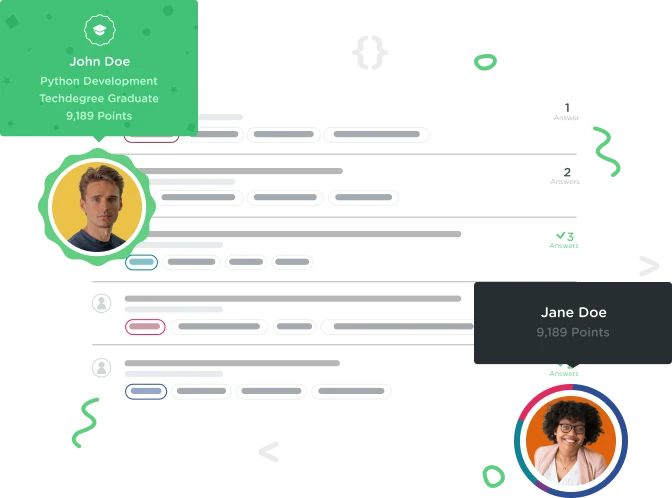
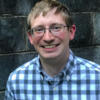
William Ray Noble
22,190 PointsIndexError: list index out of range Python OOP Basics
Hello, I'm at the end of the OOP Python Basic class which just came out. I've really enjoyed it but have hit a snag here at the end. I get an IndexError in my console.
Traceback (most recent call last):
File "game.py", line 94, in <module>
game.start_game()
File "game.py", line 82, in start_game
if self.check_match(guess1, guess2):
File "game.py", line 51, in check_match
if cards[0] == cards[1]:
IndexError: list index out of range
I've gone over the code contained in the 3.7 final video download and I can't find the problem with my check_match method that seems to be throwing the error. I have attached the rest of my code below.
class Game:
def __init__(self):
self.size = 4
self.card_options = ['Add', 'Boo', 'Cat', 'Dev', 'Egg', 'Far', 'Gum', 'Hut']
self.columns = ['A', 'B', 'C', 'D']
self.cards = []
self.locations = []
for column in self.columns:
for num in range(1, self.size + 1):
location = f'{column}{num}'
self.locations.append(location)
def set_cards(self):
used_locations = []
for card in self.card_options:
for i in range(2):
random_location = random.choice(list(set(self.locations) - set(used_locations)))
used_locations.append(random_location)
game_card = Card(card, random_location)
self.cards.append(game_card)
def create_row(self, num):
row = []
for column in self.columns:
for card in self.cards:
if card.location == f'{column}{num}':
if card.matched:
row.append(str(card))
else:
row.append(' ')
return row
def create_grid(self):
header = ' | ' + ' | '.join(self.columns) + ' |'
print(header)
for row in range(1, (self.size + 1)):
print_row = f'{row}| '
get_row = self.create_row(row)
print_row += ' | '.join(get_row) + ' |'
print(print_row)
def check_match(self, loc1, loc2):
cards = []
for card in self.cards:
if card.location == loc1 or card.location == loc2:
cards.append(card)
if cards[0] == cards[1]:
cards[0].matched = True
cards[1].matched = True
return True
else:
for card in cards:
print(f'{card.location}: {card}')
return False
def check_win(self):
for card in self.cards:
if card.matched is False:
return False
return True
def check_location(self, time):
while True:
guess = input(f"What's the location of your {time} card? ")
if guess.upper() in self.locations:
return guess.upper()
else:
print("That's not a valid location. Location should be column name then row name like A1.")
def start_game(self):
game_running = True
print("Memory Game")
self.set_cards()
while game_running:
self.create_grid()
guess1 = self.check_location("first")
guess2 = self.check_location("second")
if self.check_match(guess1, guess2):
if self.check_win():
print("Congrats, you win")
self.create_grid()
game_running = False
else:
input('those cards are not a match. Press enter to continue')
print("Game Over")
if __name__ == '__main__':
game = Game()
game.start_game()
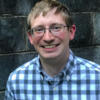
William Ray Noble
22,190 PointsTiffany Greathead, You were totally right, thank you for catching those mistakes!

Tiffany Greathead
6,613 PointsYou're welcome, I'm glad I could help!
3 Answers

Brian Heneghan
4,115 PointsHad a post typed saying "same issue here" but took some time to look through it again, and I think I cracked it.
Seemed that there was some indentation that was wrong, and it was within match_mathod, as it called out for 'cards' when it was still looking at the first card from self.cards. This incorrecr indentation is in the video I believe, so should be corrected for future videos.
So from
def check_match(self, loc1, loc2):
cards = []
for card in self.cards:
if card.location == loc1 or card.location == loc2:
cards.append(card)
if cards[0] == cards[1]:
cards[0].matched = True
cards[1].matched = True
return True
else:
for card in cards:
print(f'{card.location}: {card}')
return False
To
def check_match(self, loc1, loc2):
cards = []
for card in self.cards:
if card.location == loc1 or card.location == loc2:
cards.append(card)
if cards[0] == cards[1]:
cards[0].matched = True
cards[1].matched = True
return True
else:
for card in cards:
print(f'{card.location}: {card}')
return False

Tiffany Greathead
6,613 PointsHello,
The problem lies in the for loop inside of the check_match method:
if cards[0] == cards[1]:
cards[0].matched = True
cards[1].matched = True
return True
else:
for card in cards:
print(f'{card.location}: {card}')
return False
This section of code is included in the for loop. It needs to be outside of the for loop block in order to work.
The dashed lines aren't printing right because the "return row" section of the create_row method is indented one time too many:
def create_row(self, num):
row = []
for column in self.columns:
for card in self.cards:
if card.location == f'{column}{num}':
if card.matched:
row.append(str(card))
else:
row.append(' ')
return row
"return row" should be inline with "for column in self.columns:", otherwise it will be included in the for loop as well.
Hope this helps and makes sense. Everything else looks great.
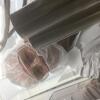
Oscar Gomez
6,797 PointsHad the same problem. Thanks for posting. Brian Heneghan's solution worked for me!
William Ray Noble
22,190 PointsWilliam Ray Noble
22,190 PointsAlso, I've noticed that the dashed line separating columns B from C, C from D, and the edge of D have disappeared even though the dashed lines on both sides of A are still present.