Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial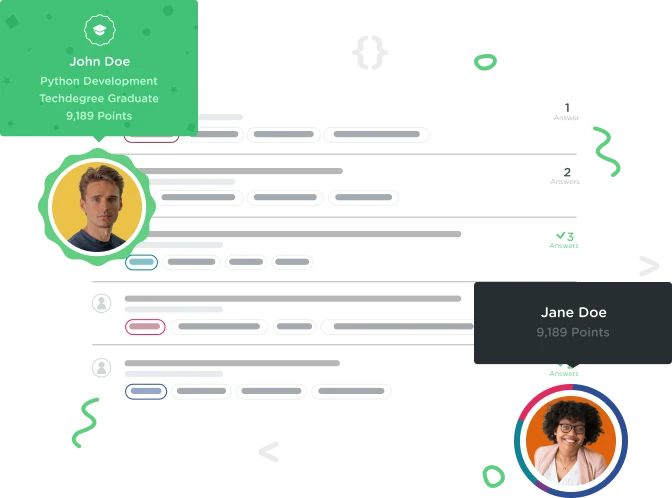
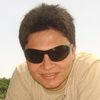
Felipe Granja
4,824 PointsIndexing Object Properties
Hi all!
I was doing some exercises online and came up with the following piece of code:
var search = function (name) {
for (var key in friends) {
if (name === friends[key].firstName) {
console.log(friends[key]);
return friends[key];
}
}
};
The code above works perfectly, but the one below doesn't:
var search = function (name) {
for (var key in friends) {
if (name === friends.key.firstName) {
console.log(friends.key);
return friends.key;
}
}
};
Can't I index object properties using both . as well as []?
Thanks in advance!
1 Answer

Leonardo Hernandez
13,798 PointsI think because your working variable 'key' is being treated as an integer by JavaScript. It works with the brackets because in that case the object property value is returned by its index.
Felipe Granja
4,824 PointsFelipe Granja
4,824 PointsWhen I was testing the code to see what I was doing wrong, I saw that the value for 'key' were the properties of the object I was inspecting.
Anyway, I just read the following on Codecademy:
"An advantage of bracket notation is that we are not restricted to just using strings in the brackets. We can also use variables whose values are property names."
http://www.codecademy.com/courses/objects-ii/0/5?curriculum_id=506324b3a7dffd00020bf661
I guess that's the reason then since 'key' is just a variable to a property name.