Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial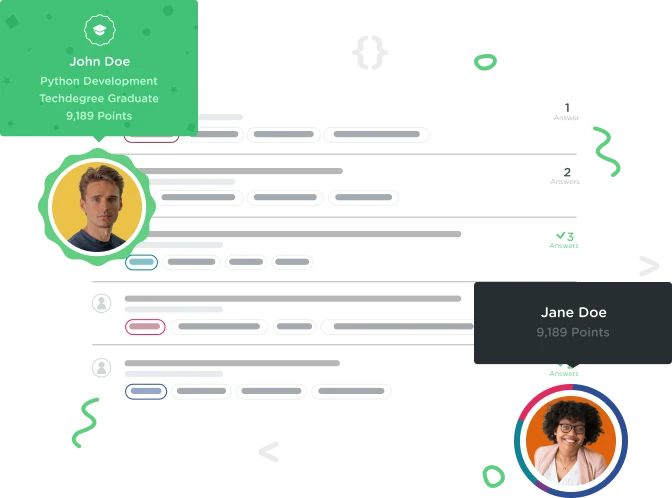

Diego Villaseñor
12,615 PointsindexOf("o")
In a code such as this
var HelloWorld = "Hello World!";
var index = HelloWorld.indexOf("o");
console.log(index);
Why is it that the console returns "4", and not "6", or "4, 6", or something like that?
2 Answers
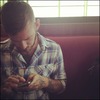
Erik McClintock
45,783 PointsDiego,
The "indexOf" function in JavaScript searches a given string for the first instance of the passed in target (or "searchValue"). Optionally, "indexOf" accepts a second parameter, which is for an offset (or "fromIndex"). Thus, when you write:
var HelloWorld = "Hello World!";
var index = HelloWorld.indexOf("o");
console.log(index);
The indexOf() function is going to scan through your HelloWorld variable (starting at the beginning of the string, because you left out the optional "fromIndex" parameter) until it reaches the first confirmed instance of the passed in searchValue, which in this case is the "o" located at the end of the word "Hello" in your string. If you recall, arrays in JavaScript (and, in this case, the string is being treated as an array of letters) are what's known as "zero indexed", meaning that the first item in the array is not positioned at index #1, but rather at index #0. Also keep in mind that every character within the string receives an index, so spaces and punctuation will also count. With that knowledge recalled, we can count the letters in the string (i.e. the items in the array) to see why the console logged 4:
H = 0
e = 1
l = 2
l = 3
o = 4
The reason that it doesn't return "6" or "4,6" is two-fold:
1) the second "o" is located at index #7, not #6, so it wouldn't return 6 anyway, but more importantly...
2) ...because the indexOf() function is designed to find the first instance of the searchValue and return its index, then stop. If you want it to continue, you've got to include the second parameter (the "fromIndex") and then add a conditional to update that offset as you find each instance of the searchValue in your string. See below:
var HelloWorld = "Hello World!"
var oIndex = HelloWorld.indexOf("o");
//as long as our searchValue is found (i.e. greater than -1)...
while ( oIndex > -1 ) {
//log the current index of the searchValue...
console.log( oIndex );
//update the starting position (or the "fromIndex", which is held in our "oIndex" variable) and continue the scan from there
//and MAKE SURE you increase the oIndex by 1, otherwise you'll be stuck in an infinite loop of it putting out the same number
//(i.e. it will start the scan at the index that it found the searchValue at, print out that it found the search value again there,
//then start from there again, then print it again, etc. etc.)
oIndex = HelloWorld.indexOf( "o", oIndex + 1 );
}
The above code will output 4 and 7 to the console.
And here is another example that will further emphasize how the oIndex gets increased and the scan continues each time we come across the searchValue:
var sentence = "Boo on you, Mr. Magoo! How dare you?!";
var oIndex = sentence.indexOf( "o" );
while( oIndex > -1 ) {
console.log( oIndex );
oIndex = sentence.indexOf( "o", oIndex + 1 );
}
The above code will output 1, 2, 4, 8, 19, 20, 24, and 33 to the console.
Official documentation is always a little dense, but here is more reading if you want to dig deeper or see more examples. Hopefully this has helped to clear things up a bit.
Happy coding!
Erik

Diego Villaseñor
12,615 PointsNice, thank you Erik!