Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial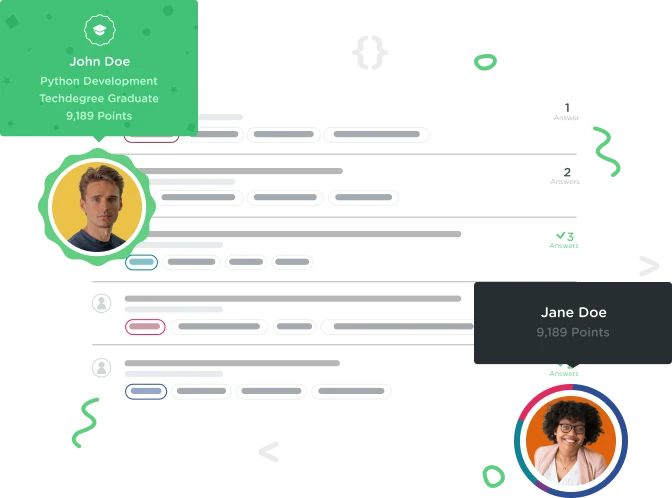

nope
Courses Plus Student 4,771 PointsIndexRoute does not work
Hi, IndexRoute does not work for me.
Here is my router code.
import React from 'react'
import { render } from 'react-dom'
import {Router, Route, IndexRoute, browserHistory} from 'react-router'
// Components
import App from './components/App'
import Home from './components/Home'
import About from './components/About'
import Teachers from './components/Teachers'
import Courses from './components/Courses'
import HTML from './components/courses/HTML'
import CSS from './components/courses/CSS'
import JavaScript from './components/courses/JavaScript'
const routes = (<Router history={browserHistory}>
<Route component={App}>
<Route path='/' component={Home} />
<Route path='about' component={About} />
<Route path='courses' component={Courses} />
<Route path='teachers' component={Teachers} />
<Route path='courses' component={Courses}>
<IndexRoute component={HTML} />
<Route path='html' component={HTML} />
<Route path='css' component={CSS} />
<Route path='javascript' component={JavaScript} />
</Route>
</Route>
</Router>)
export default routes
Can anyone spot the errors? Same problem with IndexRedirect: /courses just displays the buttons (however the routes are workign)
Kristóf Dombi
28,047 PointsKristóf Dombi
28,047 PointsHey nope!
Quick fix maybe: Try using
Redirect
component instead ofIndexRoute
.If that didn't work: I guess you use the older version of react-router, i use the newer ones, so lots of stuff was different from the videos. I hat to read the manual to get this working. Anyways, here is my solution:
router.js
App.js
Courses.js
Hope I could help! :)