Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial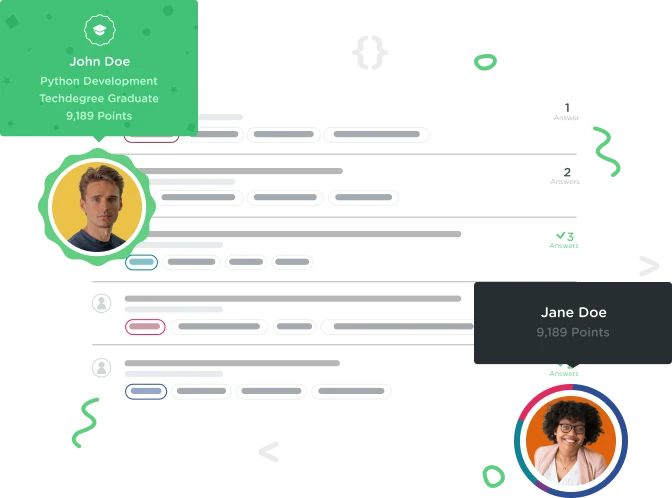

ixrevo
32,051 PointsInexplicably cannot pass 2nd task in the challenge "Using stripos: Starts with R"
Hi everyone!
The first step of this challenge I've passed easy.
Task 1, Initial code:
<?php
$var1 = "Broccoli";
$var2 = "Cream Soda";
$initial = "R";
if (stripos($var1,$initial) == 1 && stripos($var2,$initial) == 1) {
echo $var1 . " and " . $var2 . " both start with " . $initial . ".";
} else {
echo "Either " . $var1 . " or " . $var2 . " doesn't start with " . $initial . "; maybe neither of them do.";
}
?>
Task 1, code that passes the challenge:
<?php
$var1 = "Broccoli";
$var2 = "Cream Soda";
$initial = "R";
if (strpos($var1,$initial) == 1 && strpos($var2,$initial) == 1) {
echo $var1 . " and " . $var2 . " both start with " . $initial . ".";
} else {
echo "Either " . $var1 . " or " . $var2 . " doesn't start with " . $initial . "; maybe neither of them do.";
}
?>
Task 2: The code is now displaying the right message. Thanks! Let’s change both variables to words that do start with R to make sure the code still displays the right message: change $var1 to Rocky Road and $var2 to Raspberry.
I've done exactly what the task objective says:
<?php
$var1 = "Rocky Road";
$var2 = "Raspberry";
$initial = "R";
if (strpos($var1,$initial) == 1 && strpos($var2,$initial) == 1) {
echo $var1 . " and " . $var2 . " both start with " . $initial . ".";
} else {
echo "Either " . $var1 . " or " . $var2 . " doesn't start with " . $initial . "; maybe neither of them do.";
}
?>
But instead of passing of the challenge I see the following error message: Oops! It looks like Task 1 is no longer passing.
What am I doing wrong?
3 Answers
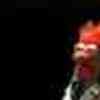
James Andrews
7,245 Pointsthe Rs are at position 0 not position 1 you need to test against position 0
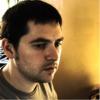
geoffrey
28,736 PointsI've just passed these exercises and In fact I noticed that if you replace directly the value inside the variable by the ones they asked you to put, that doesn't work. Instead, I defined again the variables with the new variables and then it passed.
<?php
$var1 = "Broccoli";
$var2 = "Cream Soda";
$var1 = "Rocky Road";
$var2 = "Raspberry";
$initial = "R";
?>
I did this for each one and I could pass all the exercises successfully.
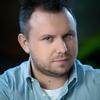
Marcin Robert Kaźmierczak
33,570 Points<?php
$var1 = "Rocky Road";
$var2 = "Raspberry";
$initial = "R";
if (strpos($var1,$initial) == 0 && strpos($var2,$initial) == 0) {
echo $var1 . " and " . $var2 . " both start with " . $initial . ".";
} else {
echo "Either " . $var1 . " or " . $var2 . " doesn't start with " . $initial . "; maybe neither of them do.";
}
?>
James Andrews
7,245 PointsJames Andrews
7,245 PointsOr I could say that the message that you are getting is exactly what you should be getting with Rs at the 0 place instead of the 1 place with the previous words.
ixrevo
32,051 Pointsixrevo
32,051 PointsYep, I've already figured out that I got the first task of this challenge wrong.
Thank you in any way!