Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial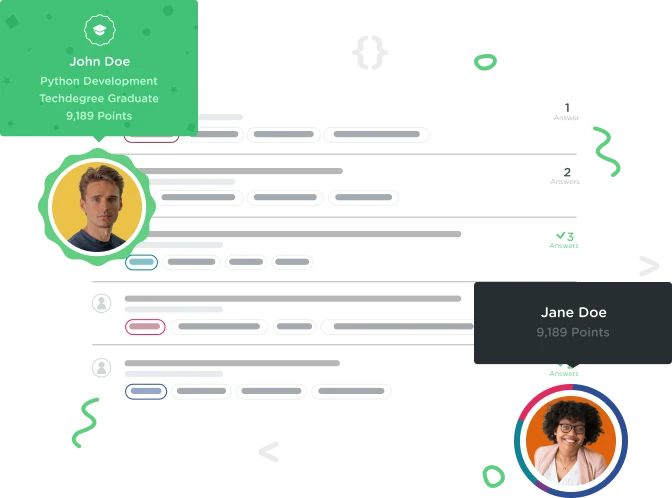
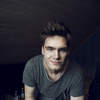
David Karlsson
4,180 PointsInherit functions?
What if you wanted to add a single row to the toHTML function? Is it possible to make Movie and Song call the same function but with small tweaks? Like, just add a line at the end of the movie but still take the title and duration of "this" for both Song and Movie? If that makes any sense to anyone..
Instead of copying the entire thing and pasting it into both functions with small tweaks.
2 Answers
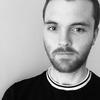
Nick Field
17,091 PointsHey David,
Just to make the programming a bit more DRY, you could use Ognjen's function as a prototype for the Media function like so;
Media.prototype.toHTML = function() {
var htmlString = '<li'
if ( this.isPlaying ) {
htmlString += ' class="current"';
}
htmlString += '>';
htmlString += this.title;
htmlString += ' - ';
if ( !this.artist ) {
htmlString += ' (';
htmlString += this.year;
htmlString += ') ';
} else {
htmlString += this.artist;
}
htmlString += '<span class="duration">';
htmlString += this.duration;
htmlString += '</span></li>';
return htmlString;
};
and completely remove the .toHTML functions in both the song.js file and the movie.js file, and the program will function the same, as both Song and Movie functions will inherit this Media prototype.
Hope that helps too :)

Ognjen Jevremovic
13,028 PointsHello David, how are you? I think I understand what you're trying to accomplish with your code. Heck, even I thought of the same, when I watched for these videos for the 2nd time.
Here's how approached it:
There's only one conditional
statement that needs to be added to the code and it looks like this:
if ( !this.artist ) {
htmlString += '( ';
htmlString += this.year;
htmlString += ' )';
} else {
htmlString += this.artist;
}
Now, what this code will do - it will check if the actual value associated with the artist
property is falsey.
In JavaScript programming language we call values like :
null,
undefined,
NaN (Not a number),
"" (empty strings),
false
== > Falsey values.
In the code we've added, we're just checking if the artist is undefined; in other words, is there even an artist
property in our object (meaning, is it even defined with a value).
The only thing that can be a bit confusing is if the conditional statement is true. Because we're checking if the value is falsey (in our example if the value returned is undefined
) that means that the value is falsey and yes the condition is true!
If it's still a bit confusing, we could rewrite the code we've added, to look like this:
if ( this.artist === undefined ) {
htmlString += '( ';
htmlString += this.year;
htmlString += ' )';
} else {
htmlString += this.artist;
}
It does the exact same thing, but it's not so flexible; you can think of the as the difference on where should we use ==
operator and where the ===
operator should be used.
It's more of a best practice I'd say.
Hope that helps! Cheers
*** Update :
I forgot to mention that falsey
values are actualy checked by including !
(exclamation mark) before the actual value.
if ( !undefined ) {
console.log( "It's falsey!" );
} else {
console.log( "The value is not falsey" );
}
//See the ! just before undefined value? That's the operator for checking the falsey(ness) of value.
Happy coding!
TL:DR
Song.prototype.toHTML = function() {
var htmlString = '<li'
if ( this.isPlaying ) {
htmlString += ' class="current"';
}
htmlString += '>';
htmlString += this.title;
htmlString += ' - ';
if ( !this.artist ) {
htmlString += ' (';
htmlString += this.year;
htmlString += ') ';
} else {
htmlString += this.artist;
}
htmlString += '<span class="duration">';
htmlString += this.duration;
htmlString += '</span></li>';
return htmlString;
};
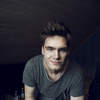
David Karlsson
4,180 PointsHm, yeah, makes sense. I thought there'd be something built in that was inheritance of functions, but this works too I guess. :)
Ashi A
2,531 PointsAshi A
2,531 PointsI was thinking about the same thing when I followed along with Andrew's example. :)