Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial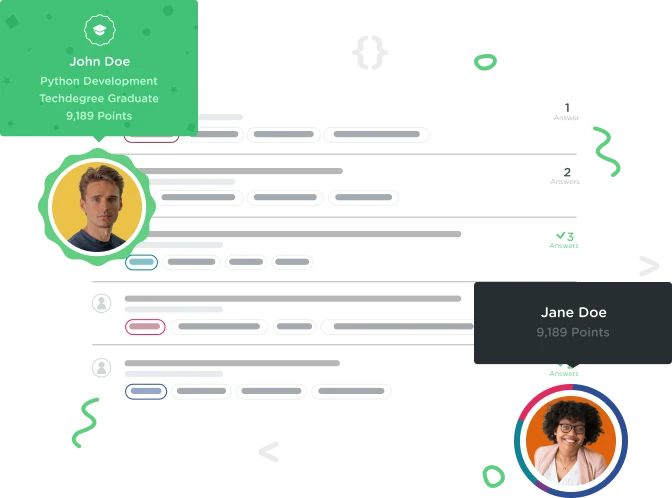

NATHAN TRAN
2,367 PointsInheritance
I got the Class properties down but I do not know what I did to the constant "someCar". What is wrong?
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
// Enter your code below
class Car: Vehicle {
var numberOfSeats = 4
}
let someCar = Car()
5 Answers
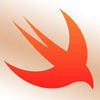
Jeff McDivitt
23,970 PointsHi Mikis/Nathan -
Mikis is correct a further explanation would have suited you better for the challenge
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
class Car: Vehicle {
// You are declaring the new variable numberOfSeats
var numberOfSeats: Int
//You need to use override init to ovveride the current initialization
override init(withDoors doors: Int, andWheels wheels: Int) {
//You will need to then initialize number of seats
self.numberOfSeats = 4
//You can call super.init after the properties of the subclass have been initialized
super.init(withDoors: 4, andWheels: 4)
}
}
//You create and an instance and assign it to a let constant someCar
let someCar = Car(withDoors: 4, andWheels: 4)
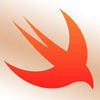
Jeff McDivitt
23,970 PointsHi Nathan - You are overthinking this one. There is not need to create an override init or super.init. The class Car inherits from Vehicle. The below is all you need; let me know if that makes sense
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
class Car: Vehicle {
var numberOfSeats: Int = 4
}
let someCar = Car(withDoors: 2, andWheels: 3)
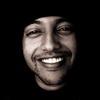
miikis
44,957 PointsHey Jeff,
There's no point in taking a course, or passing a Challenge, if you don't learn anything in the process. This Challenge was designed to test one's understanding of the concept of Inheritance in Swift. Obviously, Nathan is still learning the ins-and-outs of this subject. All of which is to say that you're doing no one a service by simply posting the answer to the Challenge, especially an answer that avoids the issue of inheritance almost completely.

NATHAN TRAN
2,367 PointsMikis
Thanks for the advice and help. I do look at the answer and go back to read redo and read the materials. With Jeff's answer, I know it is the easy answer but I do look to figure out your method too. There are more than on answer. Thank Mikis and Jeff.
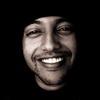
miikis
44,957 PointsHey Nathan,
You want that numberOfSeats to be configurable, at least the Challenge wants it that way. This means you'll have to create a custom initializer for Car that takes doors, wheels and numberOfSeats as arguments. Let me know if that clears it up.

NATHAN TRAN
2,367 PointsI wrote up the "seats" part assuming that doors and wheels are properties that can be use from the initial "Vehicle" class. But it is not correct, something is wrong; I tried it on xCode and the complier give me this:
****"self.numberOfSeats = seats" ==>Super.init isn't called on all paths before returning from initializer
class Car: Vehicle {
var numberOfSeats: Int
init(withSeats seats: Int) {
self.numberOfSeats = seats****
}
}
let someCar = Car(withSeats: 4)
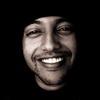
miikis
44,957 PointsSo, when you create a class (B) by inheriting from a class (A), you need to initialize all the stored properties of the class (B), as well as all the stored properties of the class (A). In other words, your class B will need to call super.init(...whatever...) at some point after initializing all of its own stored properties. In this particular case, this means Car will need to call super.init(withDoors: doors, andWheels: wheels) at some point after initializing its own numberOfSeats property. Feel free to keep asking until it makes sense :)

NATHAN TRAN
2,367 PointsIs the "super.init" section correct?
class Car: Vehicle {
var numberOfSeats: Int
==> super.init(withDoors doors: Int, andwheels wheels: Int, withSeats seats: Int) {<==
self.numberOfSeats = seats
}
}
let someCar = Car(withDoors: 4, andwheels: 4, withSeats: 4)
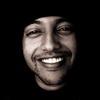
miikis
44,957 PointsAlmost. But that's not the correct syntax. You have to create a new init()
method, or override the existing init(withDoors: Int, andWheels: Int)
method. And then —inside of the init() method — you call your super.init(...stuff...)... after you initialize numberOfSeats.

NATHAN TRAN
2,367 PointsI got to this point; the only thing the complier says is "unexpected separator" (**) at "doors".
class Car: Vehicle {
var numberOfSeats: Int
override init(withDoors doors: Int, andWheels wheels: Int) {
super.init(withDoors **doors: Int, andWheels wheels: Int, andSeats seats: Int) {
self.numberOfSeats = 4
}
}
let someCar = Car(withDoors: 4, andWheels: 4)
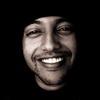
miikis
44,957 Points- You have two asterisks right before the local-parameter named doors. Probably a typo.
- When you call super.init(), you have to pass in the actual values... as opposed to what you're doing which is passing in an Int data-type. That is, you have to replace Int with actual integers. Easy fix, though. You can just use the doors and wheels values that are passed into your overridden init method.
- You're initializing numberOfSeats after you call super.init(...); you have to initialize numberOfSeats before calling super.init(...).
NATHAN TRAN
2,367 PointsNATHAN TRAN
2,367 PointsJeff or Mikis:
I have a few questions below for this code challenge:
class car: Vehicle {
// why do we have to super.init "withDoors: 4, andWheels: 4" and also added down to "Car(withDoors: 4, andWheels: 4)" } }
let someCar = Car(withDoors: 4, andWheels: 4)
class Car: Vehicle { var numberOfSeats: Int = 4 } let someCar = Car(withDoors: 2, andWheels: 3)
// so this one is the short version of the above codes? Why doesn't it needs the override and super.init?
Thanks guys