Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial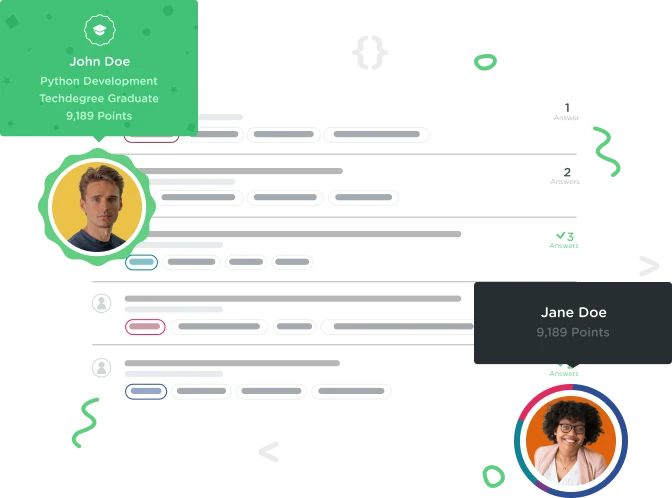

Travis John Villanueva
5,052 PointsInheritance- initializing the base class
I think i did my best, can somebody help me spit my error?
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square: Polygon
{
public readonly int SideLength;
public Square(int sideLength): base(int numSides)
{
Square square = new Square (4);
}
}
}
3 Answers

Travis John Villanueva
5,052 PointsThanks tree House Community. It is sinking in to me now :) Shout out to Luke, Alexander and Ford!

Alexander La Bianca
15,959 PointsHello,
You are calling the Square constructor in the Square constructor. Which is why this is not passing
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square: Polygon
{
public readonly int SideLength;
//You got everything correct up to this point
//You want to create the Square constructor as follows:
//you want to give the square constructor a parameter for sideLength AND every time you call the square constructor
//automatically call the Polygon Construcotr 'Polygon(int numsides)' since a square always has 4 sides, you enter 4.
//all 'base(4) does is -> Polygon(4) to the same time you call the Square constructor
public Square(int sideLength): base(4)
{
SideLength = sideLength;
}
}
//you could also create a triangle the same way
class Triangle: Polygon
{
public readonly int SideLength;
public Triangle(int sideLength):base(3)
{
SideLength = sideLength
}
}
}
Square mySquare = new Square(5); //a square with 5 sides
mySquare.SideLength // will return 5
mySquare.numsides //will return 4
Triangle myTriangle = new Triangle(5);
myTriangle.SideLength //will return 5
myTriangle.numsides //will return 3
Hope this makes a bit of sense understanding this

luke hammer
25,513 Pointsok you are a lot closer in this example vs. other question.
on the line
public Square(int sideLength): base(int numSides)
you do not need the type on the base class. and this is where you define the number of sides.
thing of the area right after the ":" in
public Square(int sideLength): base(int numSides)
as calling the parent constructor.
inside the constructor for Square you should only be working with the SideLength.
the number of sides is defined in the call to the base classes.