Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial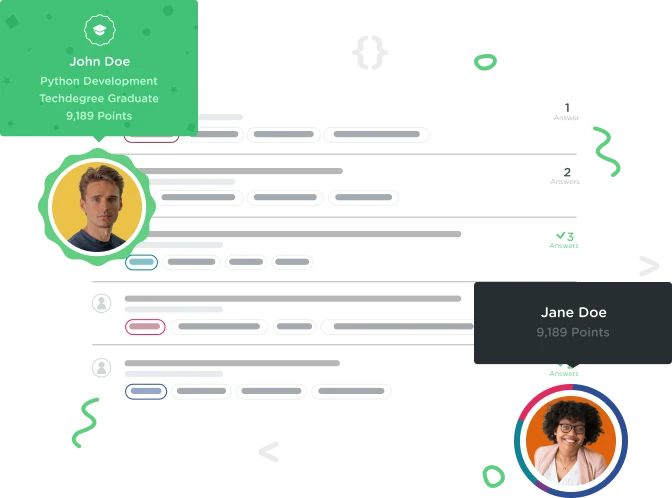

Stefano Nebiolo
1,991 Pointsinheritance with super()
Hello everybody. I thought I understood the inheritance and super function but after few more exercises, I am a bit lost. Defining a subclass of a superclass, the subclass inherits all the attributes and methods of the superclass. Then if I need to override the method within the subclass, adding or modifying something, I use the super() function. Then why in an example of the following I do not use the super() function and all the methods defined in my Vehicle class work perfectly when instantiating the subclass Car, while in the example below it doesn't?
'from abc import ABCMeta, abstractmethod
program to sell/buy vehicles
class Vehicle(object):
__metaclass__ = ABCMeta
base_sale_price = 0
wheels = 0
def __init__(self, miles, make, model, year, sold_on):
self.miles = miles
self.make = make
self.model = model
self.year = year
self.sold_on = sold_on
# set sale price as 5000 euro per wheel in the vehicle
def sale_price(self):
if self.sold_on is not None:
return 0.0 # the car is sold
return 5000.0 * self.wheels
# sef purchase as the sell price per vehicle minus 10% of the used miles
def purchase_price(self):
if self.sold_on is None:
return 0.0 # the car is still in our shop (not yet sold)
return self.base_sale_price - (.10*self.miles) # here we do have the only data different between classes: base_sale_price
@abstractmethod
def vehicle_type(self):
"""Return a string representing the type of vehicle this is."""
pass
class Car(Vehicle): """A car for sale by the shop."""
base_sale_price = 8000.0
wheels = 4
def vehicle_type(self):
"""Return a string representing the type of vehicle this is."""
return 'car''
'class Inventory: def init(self): self.slots = []
def add_item(self, item=""):
self.slots.append(item)
class SortedInventory(Inventory):
def show(self):
print(self.slots)
a = SortedInventory a.add_item(item='car') a.show()'
When I call this program, the answer I get is TypeError: show() missing 1 required positional argument: 'self' Shouldn't the add_item method works within the subclass as well since it is defined in the superclass?
1 Answer
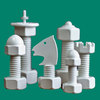
Steven Parker
231,269 PointsIt's a bit tricky to read without code formatting, but if "a" is intended to be an instance of "SortedInventory", then there should be a pair of parentheses after the class name:
a = SortedInventory()
When posting code, use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.
Stefano Nebiolo
1,991 PointsStefano Nebiolo
1,991 PointsI am sorry for the format. Here the code:
Ex1)
Ex2)
Steven Parker
231,269 PointsSteven Parker
231,269 PointsEven after formatting, it looks like the same thing. The code has "
a = SortedInventory
" (which copies the class itself) instead of "a = SortedInventory()
" (which creates a class instance).