Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial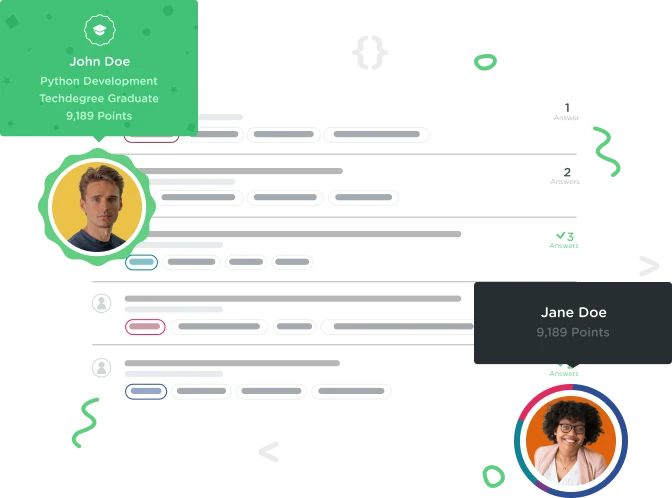

Matt Conway
1,572 PointsInit method
I am not sure what to put inside the init method!
Challenge Task 1 of 1
Let's get in some practice creating a class. Declare a class named Shape with a variable property named numberOfSides of type Int.
Remember that with classes you are required to write an initializer method.
Once you have a class definition, create an instance and assign it to a constant named someShape.
/Users/mattconway/Desktop/Screen Shot 2016-01-23 at 5.03.08 PM.png
// Enter your code below
class Shape {
var numberOfSides: Int
init(x: Int, y: Int) {
}
let someShape = Shape(x: Int, y: Int)
}
4 Answers
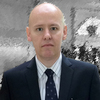
Nathan Tallack
22,160 PointsVery close. You just need to set that numberOfSides property inside your method. We do that by referring to it with the self. prefix. We also need to give it some values. For your example I am using 4 as the number of sides.
You also need to pass in some values when you are declaring your someShape. For your example I am using 2 and 2. :)
Your code would look like this.
class Shape {
var numberOfSides: Int
init(x: Int, y: Int) {
self.numberOfSides = 4
}
}
let someShape = Shape(x: 2, y: 2)

Matt Conway
1,572 PointsYou were right, it was a typo mistake that I made, so now everything is ok. Thanks for your help

Dale Bailey
20,269 PointsYou do not need x & y, please see my answer

Dale Bailey
20,269 PointsHi Nathan Tallack's answer is wrong, I don't know why people are trying to pass in x & y as it did not ask for them, it is not necessary. Define a class called "Shape", create an Int var called "numberOfSides", initialise it and then assign and instance of "Shape" to a constant called "someShape"
class Shape {
var numberOfSides : Int
init(numberOfSides: Int) {
self.numberOfSides = numberOfSides
}
}
let someShape = Shape(numberOfSides: 8)

Steven Ossorio
1,706 PointsHey Dale, Thanks for your explanation. It's true, nothing was asked in regards to x and y. Just a heads up, your variable has a little issue. I know you meant var numberOfSides: but just letting you.
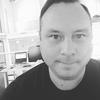
jeremiahjacquet
16,885 PointsDale Bailey Your solution is the correct way as it does not specify x and y values. The only issue i am getting is you cannot assign the the property to itself 'self.numberOfSides = numberOfSides'
I added an int as the an initial value and got no errors in the playground.
class Shape {
var numberOfSides: Int
init(numberOfSide: Int) {
self.numberOfSides = 8
}
}
let someShape = Shape(numberOfSide: 6)

Matt Conway
1,572 PointsI typed exact code you listed in Playgrounds, got 3 errors. Also typed in Challenge, got a whole bunch of errors!! Here is what it said:
class Shape Int { var numberOfSides: Int init(x: Int, y: Int) { self.numberOfSides = 4 } } let someShape = Shape(x: 2, y: 2)
swift_lint.swift:4:13: error: expected '{' in class class Shape Int { ^ swift_lint.swift:4:17: error: statement cannot begin with a closure expression class Shape Int { ^ swift_lint.swift:4:17: note: explicitly discard the result of the closure by assigning to '_' class Shape Int { ^ _ = swift_lint.swift:6:7: error: initializers may only be declared within a type init(x: Int, y: Int) { ^ swift_lint.swift:4:17: error: braced block of statements is an unused closure class Shape Int { ^ swift_lint.swift:4:17: error: expression resolves to an unused function class Shape Int { ^ swift_lint.swift:10:26: error: expected ',' separator let someShape = Shape(2: Int, 2: Int) ^ , swift_lint.swift:10:26: error: expected expression in list of expressions let someShape = Shape(2: Int, 2: Int) ^ swift_lint.swift:10:26: error: expected ',' separator let someShape = Shape(2: Int, 2: Int) ^ , swift_lint.swift:10:34: error: expected ',' separator let someShape = Shape(2: Int, 2: Int) ^ , swift_lint.swift:10:34: error: expected expression in list of expressions let someShape = Shape(2: Int, 2: Int) ^ swift_lint.swift:10:34: error: expected ',' separator let someShape = Shape(2: Int, 2: Int) ^ ,
Dale Bailey
20,269 PointsDale Bailey
20,269 PointsYou do not need x & y, please see my answer