Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial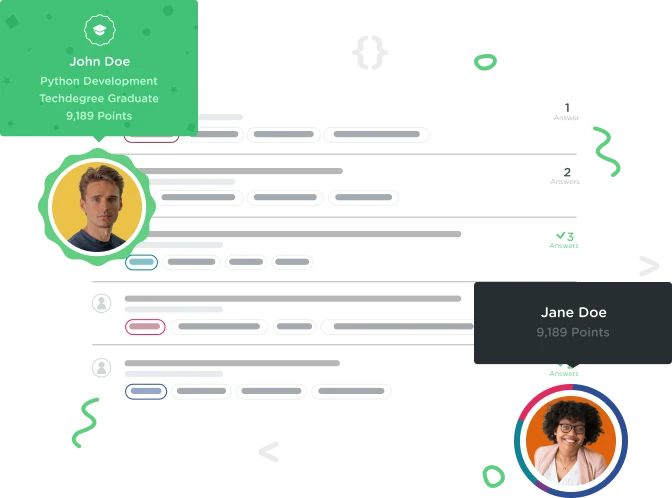
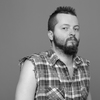
Ivan Dergachev
4,337 PointsInitialization problem when trying to get Celsius value
Hi. Here in Russia we use metric system, so I'm trying to format the temperature properly before applying it to the label in the next few steps. I've wrote a small function to convert from Fahrenheit to Celsius.
func convertToCelsius(tempFahr: Int) -> Int {
return (tempFahr - 32) * 5/9
}
And I'm calling this function during initialisation.
let tempFahrenheit = currentWeather["temperature"] as Int
temperature = convertToCelsius(tempFahrenheit)
But Xcode gives me compiler errors no matter what I try.
"Variable 'self.temperature' used before being initialized"
Here's the full code
import Foundation
import UIKit
struct Current {
var currentTime: String?
var temperature: Int
var humidity: Double
var precipProbability: Double
var summary: String
var icon: UIImage?
init(weatherDictionary: NSDictionary) {
let currentWeather = weatherDictionary["currently"] as NSDictionary
let tempFahrenheit = currentWeather["temperature"] as Int
temperature = convertToCelsius(tempFahrenheit)
humidity = currentWeather["humidity"] as Double
precipProbability = currentWeather["precipProbability"] as Double
summary = currentWeather["summary"] as String
let currentTimeIntValue = currentWeather["time"] as Int
currentTime = dateStringFromUnixTime(currentTimeIntValue)
let iconString = currentWeather["icon"] as String
icon = weatherIconFromString(iconString)
}
func dateStringFromUnixTime(unixTime: Int) -> String {
let timeInSeconds = NSTimeInterval(unixTime)
let weatherDate = NSDate(timeIntervalSince1970: timeInSeconds)
let dateFormatter = NSDateFormatter()
dateFormatter.timeStyle = .ShortStyle
return dateFormatter.stringFromDate(weatherDate)
}
func weatherIconFromString(stringIcon: String) -> UIImage {
var imageName: String
switch stringIcon {
case "clear-day":
imageName = "clear-day"
case "clear-night":
imageName = "clear-night"
case "rain":
imageName = "rain"
case "snow":
imageName = "snow"
case "sleet":
imageName = "sleet"
case "wind":
imageName = "wind"
case "fog":
imageName = "fog"
case "cloudy":
imageName = "cloudy"
case "partly-cloudy-day":
imageName = "partly-cloudy"
case "partly-cloudy-night":
imageName = "cloudy-night"
default:
imageName = "default"
}
var iconImage = UIImage(named: imageName)
return iconImage!
}
func convertToCelsius(tempFahr: Int) -> Int {
return (tempFahr - 32) * 5/9
}
}
2 Answers

dungeonkeeperhf
3,272 PointsYou are trying to assign the conversion to 'temperature', temperature is not a variable, it's the value you get from the api, the value of temperature is stored in temp farenheight.
Do this instead:
let tempFahrenheit = currentWeather["temperature"] as Int
var newTemp = convertToCelsius(tempFahrenheit)
The value of newTemp is your converted temperature.

Andrew Rodko
27,881 PointsYou can't call a function within a struct until the variables have finished initializing. The solution would be to set your temperature as an optional (like Pasan did for currentTime)
Ivan Dergachev
4,337 PointsIvan Dergachev
4,337 PointsHi! Thank you for the reply! But even this code gives me the same error.
So. This works OK:
As far as I understand, I declare the variables, then initialize the Foundation dictionary from the JSON dictionary that I received from the API, then fill the keys with the data of appropriate type.
In the example Pasan provided (with
icon
andweatherIconFromString
) he's using exactly the same pattern I'm trying to mimic: processing dictionary data with the function and only then load it into variable that will be available later in the ViewEditor ascurrentWeather.temperature
. But this (or what I provided in the original post) gives an initialization error. What am I doing wrong?