Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial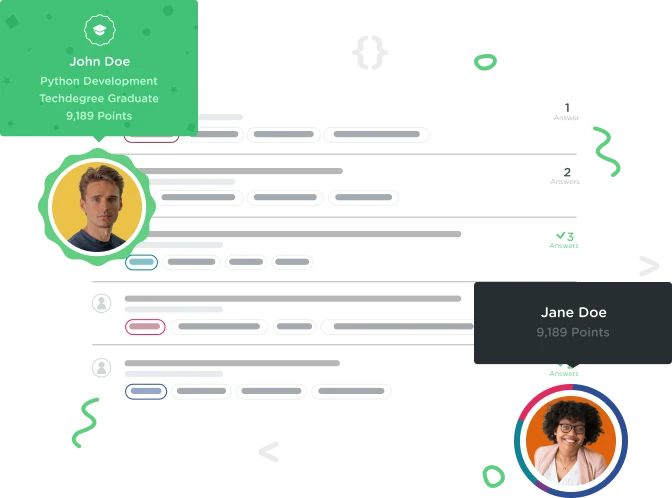
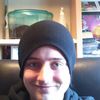
Max Reinsch
5,740 PointsInitialization- What does it mean?
I haven't seen an explanation for this.
What is it and why do we use it?
5 Answers
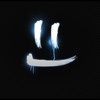
Grigorij Schleifer
10,365 PointsI had the same question a couple weeks ago and tried to summerise the topic for a better understanding. Maybe it will help you too. For the explanation I will use the link that Conor have proposed for you.
http://stackoverflow.com/questions/19941825/purpose-of-a-constructor-in-java
You have this line of code:
Bicycle myBike = new Bicycle();
Here you create a Bicycle object named myBike. This single statement performs three actions: declaration, instantiation, and initialization myBike
LEFT is the declaration side, RIGHT is the definition side !!!
Declaration:
- The code set in left associate a variable name with an object type (Bicycle).
Instantiation:
- The "new" keyword is a Java operator that creates the object
The "new" operator instantiates a class by allocating memory for a new object and returning a reference to that memory. The "new" operator also invokes the object constructor. The phrase "instantiating a class" means the same thing as "creating an object." When you create an object, you are creating an "instance" of a class, therefore "instantiating" a class.
Initialization:
- In the example above you have no parameters inside Bicycle(). So like Conor said, the new object is uninitialised at this moment. So the code above invokes the no-argument constructor. If you write your code this way;
Bicycle myBike = new Bicycle(30, 0, 8);
new Bicycle(30, 0, 8) creates space in memory for the object and initializes its fields. The constructor Bicycle() takes 30, 0 and 8 and assigns/initialises them to the object myBike. Please correct me if I am wrong. I am also a bloody beginner:)
See the complete code in the Conors link ...
Hope it helps a little bit and sorry for my bad english.
Grigorij
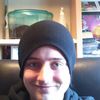
Max Reinsch
5,740 PointsRead over this again and started to grasp it (I think).
For a beginner, this a brilliant explanation.
Considered applying for a job at Treehouse? =P
Thanks Grigorij Schleifer.
Btw, your English is great!
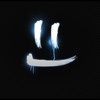
Grigorij Schleifer
10,365 PointsMax, thank you so much !!!! Never stop learning !!!

Conor Graham
7,956 PointsCorrect be if I am wrong but the reason we use initialization is so that we can use dynamic values. Now to initialize any object we have to use the a constructor . The reason we would want to initialize is so that we can use dynamic values when creating an object. Consider the example:
'''
Shape circle = new Shape(); //Here we have a new object which is uninitialised
''' Now what do we need to do to initialise it ?? Yes, thats right use a constructor.
Now what we are doing above is the following: we are creating a new object called circle. Let's just pretend we already have a Shape class created and the object circle is of the type Shape. We have initialised it through using the keyword 'new' and we have then made a call to its constructor. Now, in java every class has at least one constructor. If you did not create one then it will explicitly assign one for you. So the reason why we would want to initialise is so that we can create new objects and initialise them with an initial state or other important information about the object.
I hope you sort of half understand this. I have added a link for you to further understand here
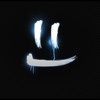
Grigorij Schleifer
10,365 PointsYou are very welcome !
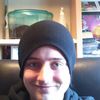
Max Reinsch
5,740 PointsThank you Conor Graham and Grigorij Schleifer.
That's a bit clearer now.
Conor Graham
7,956 PointsConor Graham
7,956 PointsIn what context? Initialization of constructors, lists,etc. Or what does it mean in general?